How to Create an ASP.NET Site Quickly (A Beginner’s Guide)
ASP.NET is a part of .NET technology used to write powerful client/server Internet applications. It allows you to create dynamic HTML pages. ASP.NET is the result of the combination of the older ASP technology (active server pages) and the .NET Framework.
It contains many ready-made controls that you can use to quickly create interactive websites. You can also use services provided by other websites.
Previously, ASP.NET technology was divided into two areas: Web Forms and Model-View-Controller (MVC). Now Microsoft developers have removed a lot of duplicate functionality, leaving a single ASP.NET Core MVC programming model.
The paradigm for building MVC applications has three components: Model, View, and Controller.
Let’s take a closer look at them:
- Model is a component of the application that is responsible for interacting with the data source (database, file system).
- View – the component responsible for displaying the user interface.
- Controller is a component that describes the application logic, in other words, the logic for processing HTTP requests to a web application. The controller interacts with model objects that affect the view.
ASP.NET Page Life Cycle
As part of this ASP.Net article, we will look at the sequence of stages of page processing:
- A page request occurs when a page is requested, the server checks to see if it is being requested the first time. If so, the page is created, the response is processed and sent to the user. If the page is not requested for the first time, the cache is checked. If the page exists in the cache, the saved response is sent to the user.
- Starting the page, at this stage, the Request and Response objects are created. The Request object is used to store information that was sent when the page was requested. The Response object is used to store information that is sent back to the user.
- Page initialization. This is where all the controls on the web page are initialized.
- Page load. The page is loaded with all defaults.
- Validation. In some cases, validation can be specified for certain forms. For example, confirmation may be requested that a list item contains a specific set of values.
- If the condition is not met, an error should be displayed when loading the page.
- Event reprocessing occurs if the page is loaded again. This happens in response to a previous event. If the user clicks on the submit button on the page, then the same page is displayed again. Then the repeated event handler is called.
- The rendering of the page occurs before the response is sent to the user. All information about the form is saved and the result is sent to the user in the form of a complete web page.
- Unloading. After the page is submitted to the user, it is no longer necessary to store the web form objects in memory. Thus, the unloading process involves removing all unnecessary objects from memory.
The internal structure of the project
After creation, the project initially already has three default pages: Default, About, and Contact.
Each page consists of three files:
Page.aspx
– Contains the HTML markup of a specific page;Page.aspx.cs
– Responsible for the logic of a specific page;Page.aspx.designer.cs
– The bridge between Page.aspx and Page.aspx.cs.
The code of the About.aspx
file:
<%@ Page Title="About" Language="C#" MasterPageFile="~/Site.Master" AutoEventWireup="true" CodeBehind="About.aspx.cs" Inherits="TutWebApplication.About" %> <asp:Content ID="BodyContent" ContentPlaceHolderID="MainContent" runat="server"> <h2><%: Title %>.</h2> <h3>Your application description page.</h3> <p>Use this area to provide additional information.</p> </asp:Content>
About.aspx
contains only a fragment of the finished page. The main part is located on the Site.Master
or Site.Mobile.Master
file.
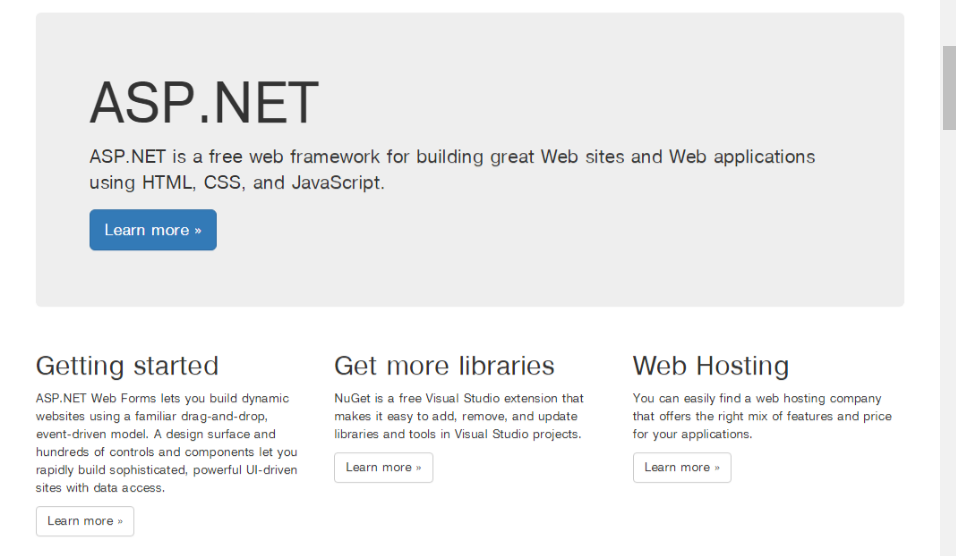
To test and run the project, press IIS Express or the traditional F5 key.
After that, all files will be compiled and the site will open at http://localhost:5000 in the default browser (the port number may differ).
How to create a page in ASP.NET Web Forms
Initially, decide in which directory you will create the pages. All files located in one directory are considered a single project. Start the development environment of your choice.
Select the File-New-Website menu item. A dialog box will appear. Assign a project name in it and select the C# programming language.
By default, the project is created on the file system. Optionally, you can create it on an HTTP or FTP server. You can also always copy a project from the file system to the server by clicking just the button "Solution Explorer".
To create other pages, right click on the project name and select Add -> Web Form from the context menu (you can take a different name for the web form, in this case a new form called “News”):
A page with the following code will be created:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="News.aspx.cs" Inherits="WebFormsApp1.News" %> <!DOCTYPE html> <html xmlns="https://www.w3.org/1999/xhtml"> <head runat="server"> <title>News</title> // Add the title of the page </head> <body> <form id="news" runat="server"> <div> Some information </div> </form> </body> </html>
Let’s take a look at this page. <%@ Page Language = "C #"%>
. The <%
tag is always intended to interpret ASP code. The Page directive is always present on the aspx page.
Its Language
attribute is an indication that the scripts for this page will use the C # programming language. CodeFile
is the name of the code-behind file. Inherits
is a class defined in this file from which the page class is inherited.
This page does not have an <asp:Content>
tag, so the template from the Site.Master
file will not be displayed. To change this, you can copy the code from About.aspx
.
How to insert HTML code into a page
To pass a string along with a tag to the HTML code, you need to use not the usual string
type, but HtmlString
:
HtmlString PageContent = new HtmlString("<p>Something!</p>");
How to add a link to the menu
The menu is located in Site.Master
:
<ul class="nav navbar-nav"> <li><a runat="server" href="~/">Home</a></li> <li><a runat="server" href="~/About">About</a></li> <li><a runat="server" href="~/Contact">Contact</a></li> <li><a runat="server" href="~/News">News</a></li> // adding link to menu </ul>
Conclusion
In this article, we have covered: creating an ASP.NET site, creating a new page, adding a link to the page in the menu, etc. To find out more information about ASP.NET, you can look at their official documentation.