How to Auto-Highlight Text Upon User Click
Some pieces of content on websites are meant to be copied by users, such as a URL address, an automatically generated API key, or a few lines of code (snippets). But copying these contents can be a challenge, particularly for users who are using a trackpad or a subpar mouse. So let’s make it easier for them.
If you have stumbled upon websites like TheNextWeb, you will find that the shortlink URL is highlighted when you click on it. Let’s check out how this is done.
Getting Started
To begin with, we lay out the HTML that wraps the shortlink URL.
<div class="shortlink"> <span class="shortlink__label">Shortlink</span> <div class="shortlink__container"> <i class="shortlink__icon ion ion-link"></i> <span class="shortlink__url">http://goo.gl/9JEpOz</span> </div> </div>
We have the URL wrapped in a span
element along with an icon from Ionicon. The style of these elements is entirely up to you, as it will depend on your website layout. But, for the purpose of this demo, I style it this way:
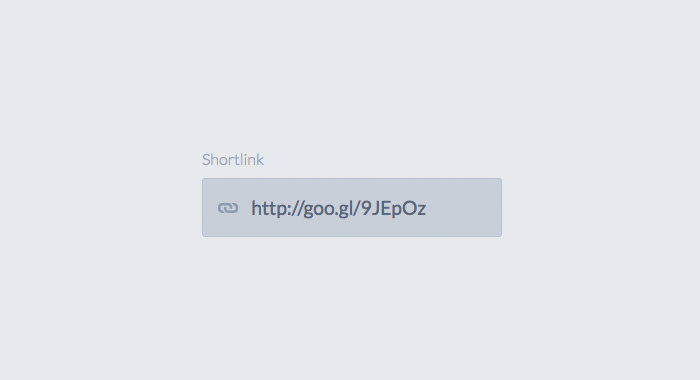
It is simple, blue and square (grab the style codes here).
The JavaScript
And here is the meat of the code, the JavaScript. The plan here is to highlight the URL as the users click on it.
We begin the code with a variable that selects the element in which the user will click.
var target = document.querySelector('.shortlink');
The querySelector
is a JavaScript method to select the element; it basically works like the jQuery constructor $()
. You can use the dot notation to get the element by the class or #
notation to get an element by the ID.
Next, we need to create a new JavaScript function.
function selection(elem) { }
We name our function as selection()
. And as you can see above, the function requires a parameter to pass the element that wraps the URL or any regular text we would like to highlight. In our case, this would be the span
element with the shortlink__url
class.
Within this function, we add a couple more variables:
var target = document.querySelector('.shortlink'); function selection(elem) { var elem = document.querySelector(elem); var select = window.getSelection(); var range = document.createRange(); }
First, the elem
variable selects the element that we pass through the parameter of the function. The second variable, select
, runs a native JavaScript function to get the selection of text. The last variable, range
controls the selection range; we would like to ensure that selection is only within the selected element.
Next, we chain these variables with a couple of other JavaScript functions as follows:
var target = document.querySelector('.shortlink'); function selection(elem) { var elem = document.querySelector(elem); var select = window.getSelection(); var range = document.createRange(); range.selectNodeContents(elem); select.addRange(range); }
The first addition, range.selectNodeContents(elem)
, defines the range of the selection which in this case is the element referred to in elem
. The last line, select.addRange(range)
makes the selection limited to the range specified.
Great! We are all set with the function. Let’s put it into action.
Run It
We bind the target element with an onclick
event. As the element is clicked, we run the function we just made and pass the parameter with the class name of the element where the URL is wrapped; in this case it is .shortlink__url
.
target.onclick = function() { selection('.shortlink__url'); };
We are done. As mentioned earlier, you can also do so for other types of content on your website which you might want your users to copy more easily.
Some of you might be wondering if we could automatically copy, instead of just highlight, the short URL upon user click. While this may seem like a really good idea, it is unfortunately not quite easy to achieve and may cause a bad user experience. The copy action should be fully under the user’s consent.
The steps in this post only take it up to the highlight action. Whether our users would copy it or not is entirely up to them.
You can follow through the following links to see the demo or download the source code.