What is OAuth Connect and How To Use It
Many of us come into contact with OAuth when browsing around the Web, and most of us aren’t even aware of its existence. OAuth(Open Authentication) is a system which grants third-party websites limited access into user accounts, for example, your Twitter or Facebook accounts.
It lets visitors interact within the site without requiring new account registration or releasing your username and password to third parties.
In this guide I’d like to introduce the concept of OAuth and how it can apply to developers. There are a lot of technical details involved in the implementation of your own OAuth application. My example will be written in PHP using a Twitter library wrapper, but you could use nearly any popular programming API from Python to Ruby or Objective-C.
Read Also: 10 HTML/CSS & JavaScript Frameworks to Build Mobile Apps
Even if the concept feels cryptic, attempt to digest as much as you can. It’s still a very mysterious technology, having just been drafted back in 2007. I certainly didn’t understand how to develop full OAuth Connections even after my first few tutorials but if you stick with it, you’ll catch on fast. Now first to kick things off, a small introduction!
What Problems Can We Solve?
If you consider how much more connected the Internet has become, it only makes sense that users will want to share information between multiple accounts from Facebook into Twitter, Tumblr, Foursquare, and now even into mobile apps such as Path or Instagram.
The problem we face now is how to accomplish this in the most secure and simplest way possible. OAuth 1.0 is an attempt to solve this and numerous other problems, compared with older OpenID standards. Users are still entering their username/password into other third party websites just to connect into OpenID.
This doesn’t make it any safer for the user. Under OAuth specs, the user never needs to store any personal account data into a third party database.
With OAuth, the main account provider (e.g. Twitter, Facebook) will first redirect you (the user) to an authorization page. The user then logs into the main network, and then either accepts or denies a new connection into the third party website.
The technology is painlessly easy and you can always unauthorize the connections from your account settings at any point. Notice that your password is never given to the 3rd party which makes this protocol a lot more safer than its counterpart.
How the Process Works
There are 3 parties to consider in a standard OAuth call:
- Service Provider – The main network you are trying to pull data from. They provide the API response such as your username, profile picture, website URL, and other stuff.
- Consumer – The 3rd party app looking to receive data. This would be the website or mobile app which makes the initial connection request, then also handles the return data after authorization.
- User – The person sitting behind the computer interacting with the websites which would be you!
The purpose of OAuth isn’t to provide a specific library for websites to use. It actually sets up the “rules” for building an open protocol API. So while we can all benefit from this technology it’s actually developers who will really find interest in this area. If you need more info check out the revised v1.0 edition released n April 2010.
Facing Security
The entire process ultimately requires 2 different keys along with an access token. The keys are provided by the root service after you register an application – these are known as your client and secret ID. The client ID is generally passed into the Authentication URL so the server can recognize your app.
The secret ID is held in your code so the server can verify your app’s identity. Similarly the remote server will match up your secret ID with their own so you don’t mistakenly send a twitter request to Facebook’s API, or vice versa.
If the user authorizes the connection and all keys match up, then they are returned to your website with a long code of random numbers and letters.
This code is used to generate a new access token. These behave similar to a session variable which you can store in a cookie to keep the user logged into your website. The only difference is that many services will send back an Access Token and Secret Access Token.
You likely need both of these to pull any data from the server. An example could be requesting the user’s profile photo to save a copy on your own website.
Example Library for Twitter OAuth
Developers aren’t often likely to start from scratch so why not look into a previously-built library? This will save us our time, and from headaches, when working with PHP. Let’s look into building a really simple example on top of the Twitter API.
I highly recommend Twitter Async by Jaisen Mathai on GitHub. It works perfectly and even provides some really straightforward example codes that we can look at. You can download the .zip for now, but before we look into the code we need to register and get our app IDs from Twitter.
Registering a New Application
The Twitter Dev Center is a great resource for those just getting started on the API. It has been written and rewritten many times over the course of a few years. The page we want is https://dev.twitter.com/apps/new. It will ask you to log in at first, then you need to enter some credentials for a new application.
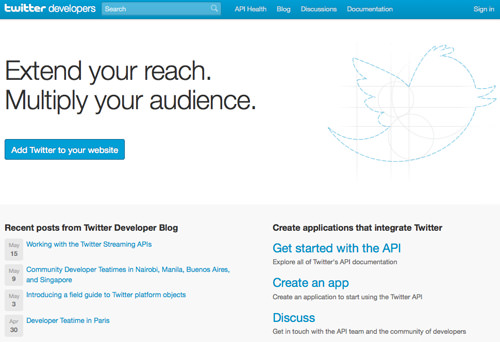
The App name and description are displayed when the user goes to authorize with Twitter. Your Web URL is also important to distinguish the third party address. It would be easier to work with a live domain although you can use localhost for testing, but I do not enourage this method. It’s just as easy to sign up for a free web host and run your scripts from there.
The Callback URL is stated as the final destination after your visitors either accept or deny the authorization. It’s your job as the programmer to read the response from Twitter and output a message accordingly.
In the Async library we’ve already got some credentials in place, but they won’t work since the callback URL is specified to an external blog. If you’re interested in building a fully-connected OAuth web app I’ve included some detailed tutorials below.
Check Out the Code
If you are using a remote web host you may want to unzip the Async libraries and upload them to a new directory. Otherwise you can just check out the source code. It’s likely that we won’t be able to pull a new connection anyways. But hands-on experience with uploading and editing source code is always a learning process.
In the root directory you’ll find a script named simpleTest.php. Inside is a whole lot of PHP codes related to the OAuth libraries included. I won’t be able to put it all together for you, but we should look at an important code block to pinpoint notable details.
<?php include 'EpiCurl.php'; include 'EpiOAuth.php'; include 'EpiTwitter.php'; $consumer_key = 'jdv3dsDhsYuJRlZFSuI2fg'; $consumer_secret = 'NNXamBsBFG8PnEmacYs0uCtbtsz346OJSod7Dl94'; $token = '25451974-uakRmTZxrSFQbkDjZnTAsxDO5o9kacz2LT6kqEHA'; $secret= 'CuQPQ1WqIdSJDTIkDUlXjHpbcRao9lcKhQHflqGE8'; $twitterObj = new EpiTwitter($consumer_key, $consumer_secret, $token, $secret); $twitterObjUnAuth = new EpiTwitter($consumer_key, $consumer_secret); ?>
There are 4 very important variables for the consumer key and secret ID, along with the token and secret token ID. Not all API services will require this set of 4, but it is proper OAuth protocol. The EpiTwitter class requires all 4 values as parameters and generates the connection URL into Twitter.
https://api.twitter.com/oauth/authorize?oauth_token=TOKEN_ID_HERE
With this new dynamic URL you can create a login button for your users. This would direct them first to a secure Twitter API page where the user either accepts or denies your connection. Regardless of their choice the user then gets re-directed to your app callback URL.
The entire open protocol has a very clean perspective which allows for rapid development, especially with libraries available in practically every language.
Related Links
- Gentle Introduction to OAuth
- OAuth FAQ
- Facebook Authentication Dev Guide
- Simple Twitter OAuth Signin
- Using OAuth with Twitter in Cocoa Objective-C
- Consuming OAuth Intelligently in Rails
Conclusion
Hopefully this introduction into OAuth has got you interested in building apps over the protocol. Many developers have been striving for just such a solution, and OAuth 2.0 may be the future of interconnected social networks. I already use over two dozen connections into my Twitter account and have been really impressed with the developer’s documentation!
Clearly there is a lot to say on this topic. It’s not something you may be able to fully process in one sitting. Browse around the net for more OAuth solutions and let us know your thoughts in the discussion area below.
Read Also: Creating Your Own Mobile App with Cordova