Getting Started with Modernizr JavaScript Library
Modernizr is a JavaScript Library used by many popular websites including Twitter, USMagazine, Good.is, About.com, etc. In one of our previous posts, we have mentioned it several times, but we have not actually dug into what Modernizr is.
So, in this post we will specifically discuss this JavaScript Library.
Getting Started with Twitter Bootstrap
Building a website from the ground up is very hard. Even some people who are able to code... Read more
What is it?
First of all, let’s get the essential question answered: what is Modernizr?
Based on the official site, Modernizr is “A JavaScript library that detects HTML5 and CSS3 features in the user’s browser.”
Although HTML5 and CSS3 are great but many of the new features they have, as we already know by now, are not much applicable in old browsers.
Modernizr, helps to address this problem by testing the user’s browser on whether it supports a particular feature. If the feature is “unsupported”, then we can deliver an appropriate script or function to imitate the feature it lacks.
Recommended Reading: What Modernizr doesn’t do?
Setting-up Modernizr
At the Modernizr official website, we will find two options to download the file, Development and Production version.
The Development version is a full and uncompressed version consisting of all the primary feature tests; while in the Production version, we can select the feature tests that we only need.
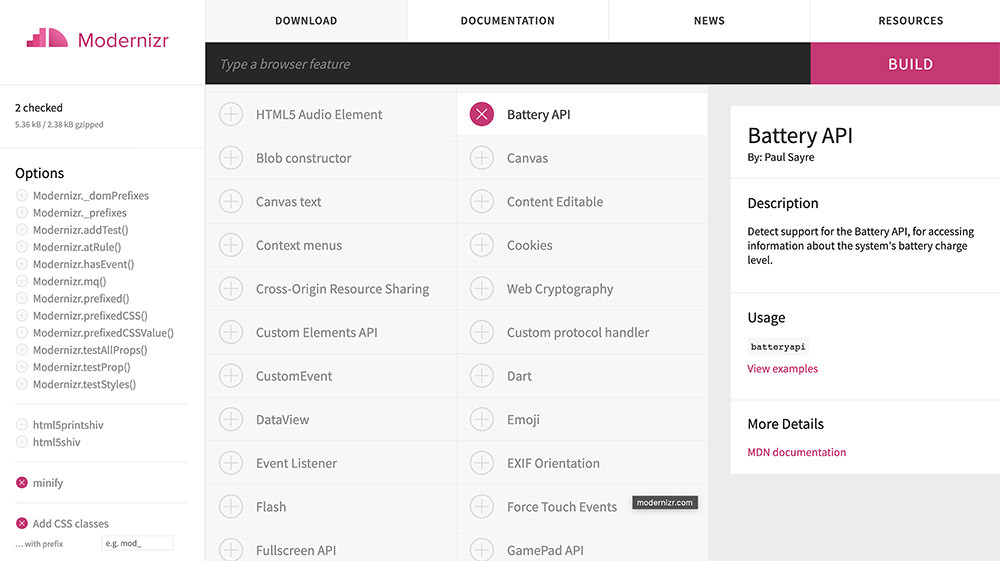
As you can see, there are a lot of options for feature tests in the download page. In this example, we will select all the primary feature tests. Generate and grab the codes. Then, insert the file inside the <head>
section.
<script src="modernizr.js" type="text/javascript"></script>
Lastly, we also need to add no-js
class to the <html>
tag.
<html class="no-js">
This class is necessary, in case the user’s browser is running without the JavaScript enabled, we can add an appropriate fallback through this class. If it does, Modernizr will replace this class with just js
.
That’s it, now we are all set up and ready to go with Modernizr.
Working with Classes
Now, if we do an Inspect Element on our webpage, you will see that there are many CSS classes added in the <html>
tag. These classes are generated from Modernizr and will vary depending on the browser’s capabilities.
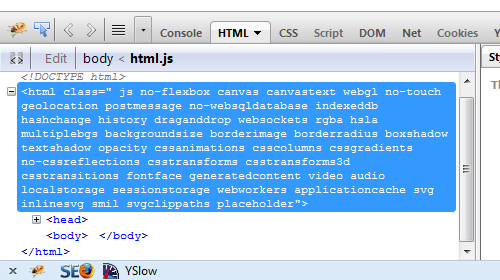
For instance, if the browser does not support CSS3 Animations, then Modernizr will generate theno-cssanimations
class.
But when it does, it will remove the no-
prefix and simply become cssanimations
.
Let’s take a look at the code snippet below;
<html class=" js no-flexbox no-canvas no-canvastext no-webgl no-touch no-geolocation postmessage no-websqldatabase no-indexeddb no-hashchange no-history draganddrop no-websockets no-rgba no-hsla no-multiplebgs no-backgroundsize no-borderimage no-borderradius no-boxshadow no-textshadow no-opacity no-cssanimations no-csscolumns no-cssgradients no-cssreflections no-csstransforms no-csstransforms3d no-csstransitions fontface no-generatedcontent no-video no-audio no-localstorage no-sessionstorage no-webworkers no-applicationcache no-svg no-inlinesvg no-smil no-svgclippaths no-placeholder">
The code above is taken from Internet Explorer 7 and as we can see from the generated classes, this browser clearly lacks in so many good new features, such as no support for CSS3 border-radius
.
So, in case we want to replace the border radius presentation, let’s say, with rounded corner images in Internet Explorer 7, then we can use the no-broderradius
class.
.no-borderradius div { /*-- do some hacks here --*/ }
If you are wondering how far your current browser is able to handle new HTML5 and CSS3 technology, Modernizr has provided a test suit you can use. Visit this page (Modernizr Test Suit), and you will instantly see the output.
A Guide to Understanding CSS Style Priorities
The Cascading Style Sheet (CSS) is one of the simplest web-related languages. Hence, many beginners starting their web... Read more
Browser Feature Test
Next, as mentioned above, Modernizr is made to detect or test browser features (easily). To test the browser feature we can use the following syntax;
Modernizr.featuretodetect
Assuming we want to test whether the browser supports WebGL we can write;
if (Modernizr.webgl) { /* Script A */ } else { /* Script B */ }
We can also negate the statement, that way the result will return the opposite; it is true
, when the browser doesn’t support the feature;
if (!Modernizr.webgl) { }
Or, if we want to run different scripts depending on the result test, we can also extend the code, as follows;
if (Modernizr.webgl) { /* Script A */ } else { /* Script B */ }
The code above states that if the browser supports WebGL then Script A will run, if it does not, then Script B will run. That way, the script will only be loaded when the condition is met and the script is actually needed.
Modernizr.load
One more thing that we are going to take a look at from Modernizr is the Modernizr.load
. Modernizr.load
is a conditional resource (CSS and JS) loader that is based on Yepnope. Let’s take a look at the following code;
Modernizr.load({ test: Modernizr.webgl, yep : 'three.js', /* JavaScript 3D Library */ nope: 'jebgl.js' /* */ });
Similar to the previous section, in the example above, we run a test to see if the user’s browser supports WebGL. If the result returns yep (it supports WebGL) then we will load three.js, but if it returns nope (it does not support WebGL) then we will load jebgl.js as the alternative.
In case we only need one condition to load the script, we can remove the other one that we don’t need. The following examples shows how we load placeme.js, if the user’s browser does not support placeholder
attribute and will load nothing when it does.
Modernizr.load({ test: Modernizr.placeholder, nope: 'placeme.js' });
This practice, as in our previous discussion, will ensure that the users will have the best load performance by avoiding unnecessary bits to download – in other words, we will only load script, only when it is needed.
For further advanced implementation of Modernizr.load
you can refer to its official documentation or you can also refer to Yepnope documentation where it is originally derived from.
Final Thoughts
Modernizr has already done the hard work and save a lot of our time for us, so there is no reason to not include this JavaScript Library when you are working with HTML5 and CSS3, particularly when you need to fill in the gap between new technologies and old browsers.