Optimizing Source Code Comments for Clarity and Efficiency
Developers working on large projects quickly learn the importance of code comments. As you build numerous features into the same application, things can become complex. With so many elements like functions, variable references, return values, and parameters, how can you keep everything organized?
It’s no surprise that commenting your code is crucial for both solo and team projects. However, many developers are unsure of the best practices for doing so. Below, I’ve shared some personal tips for crafting neat, well-formatted code comments. Standards and comment templates may vary among developers, but your goal should always be clear and readable comments to explain any potentially confusing areas in your code.
Let’s begin by discussing some of the differences in comment formatting. This will give you a better understanding of just how detailed you can be with project code. Afterward, I’ll share specific tips and examples that you can start using right away!
Comment Styles: An Overview
The ideas presented here are intended as guidelines for cleaner comments. Individual programming languages don’t typically provide specific guidelines or specifications for how to structure your documentation.
That said, modern developers have developed their own systems of code commenting. I’ll cover a few mainstream styles and detail their purposes.
Inline Commenting
Almost every programming language supports inline comments. These are limited to single-line content and only comment on the text after a certain point. For example, in C/C++, you begin an inline comment like this:
// Start variable listing var myvar = 1;
Inline comments are perfect for briefly explaining potentially confusing functionality. If you’re working with many parameters or function calls, you might place several inline comments nearby. However, the most beneficial use is a simple explanation for small functionality.
if(callAjax($params)) { // Successfully run callAjax with user parameters ... code }
In the example above, the code must be on a new line after the opening bracket; otherwise, it would all be caught on the same comment line! Avoid overusing inline comments, as you generally don’t need them on every line. However, they are helpful for particularly confusing junctions in your code and are easy to add at the last minute.
Descriptive Blocks
When a single-line comment won’t suffice, descriptive blocks come into play. These pre-formatted comment templates are widely used in programming, especially around functions and library files. Whenever you create a new function, it’s good practice to add a descriptive block above the declaration.
/** @desc Opens a modal window to display a message @param string $msg - The message to be displayed @return bool - Success or failure */ function modalPopup($msg) { ... }
In the example above, I’ve created a JavaScript function named modalPopup, which takes a single parameter. The comments above use a syntax similar to phpDocumentor, where each line begins with an @ symbol followed by a specific key. These tags, known as comment tags, are documented extensively on their website. Feel free to create your own tags and use them throughout your code as needed. They help keep everything organized so that you can quickly reference important information. I’ve used the /* */
block-style commenting format, which keeps the comments much cleaner than adding a double slash at the beginning of each line.
Group/Class Comments
Aside from commenting on functions and loops, block comments aren’t used as frequently. However, you need strong block comments at the beginning of your backend documents or library files. This practice is common in many CMSs like WordPress.
The top section of your file should contain comments regarding the file itself. This allows you to quickly identify what you’re editing when working on multiple files simultaneously. Additionally, you can use this area as a reference point for the most important functions within the class.
/** @desc This class holds functions for user interaction Examples include user_pass(), user_username(), user_age(), user_regdate() @author Jake Rocheleau jakerocheleau@gmail.com @required settings.php */ abstract class myWebClass { }
In the example above, I’ve created a sample class named myWebClass
. I’ve added meta information, including my name and email address for contact. When developers write open-source code, this is generally a good practice so that others may contact you for support. This method is also useful when working in larger development teams.
The tag @required
isn’t widely used, but I’ve maintained it in a few of my projects, particularly on pages where I’ve customized many methods. When including files in a project, they must be loaded before any code is output. Adding these details to the main class comment block is a good way to remember which files are required.
Front-end Code Commenting
Now that we’ve covered three important comment templates, let’s explore some other examples. Many front-end developers have transitioned from static HTML to jQuery and CSS. While HTML comments aren’t as critical as in programming, when writing style libraries and page scripts, things can get messy over time.
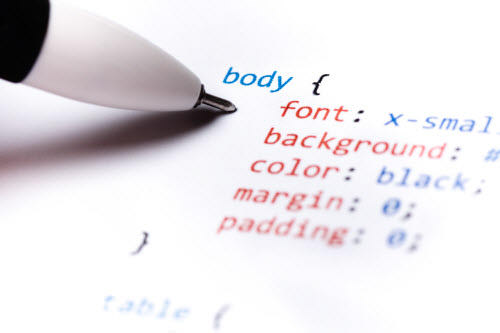
JavaScript follows a more traditional commenting method, similar to Java, PHP, and C/C++. CSS only uses block-style comments denoted by a slash and asterisk. Remember, since neither CSS nor JS is parsed server-side, comments will be visible to your visitors. However, both methods are effective for leaving helpful notes in your code to review later.
Breaking up CSS files can be tedious. We all know about leaving inline comments to explain fixes for Internet Explorer or Safari. But I believe CSS commenting can be as organized as jQuery and PHP commenting. Let’s dive into creating style groups before discussing some detailed tips for code commenting.
CSS Style Groups
For seasoned CSS designers, commenting becomes second nature. You gradually memorize all the properties, syntax, and develop your own system for stylesheets. Through my experience, I’ve developed a method called grouping to organize similar CSS blocks into specific areas.
When I need to edit CSS, I can quickly find what I need. How you choose to group styles is entirely up to you, which is the beauty of this system. Below are a few preset standards I’ve established:
- @resets – Resets default browser margins, padding, fonts, colors, etc.
- @fonts – Styles for paragraphs, headings, blockquotes, links, code
- @navigation – Main website navigation links
- @layout – Wrapper, container, sidebars
- @header & @footer – May vary based on your design. Possible styles include links, unordered lists, footer columns, headings, sub-navs
When grouping stylesheets, I’ve found the tagging system to be incredibly helpful. Unlike PHP or JavaScript, I use a single @group tag followed by a category or keywords. Below are two examples to illustrate:
/** @group footer */ #footer { styles... }
/** @group footer, small fonts, columns, external links **/
Alternatively, you could add more detail to each comment block. I prefer to keep things simple and straightforward so the stylesheets are easy to skim. Commenting is all about documentation, so as long as you understand your notes, you’re good to go!
4 Tips for Better Comment Styling
We’ve spent the first half of this article exploring various formats for code commenting. Now, let’s discuss some overall tips for keeping your code clean, organized, and easy to understand.
1. Keep Everything Readable
As developers, we sometimes forget that we’re writing comments for humans to read. All programming languages are designed for machines, so it can be tedious to translate code into plain, written text. It’s important to remember that you’re not writing a research paper – you’re simply leaving helpful tips!
function getTheMail() { // Code here will build e-mail // Run code if our custom sendMyMail() function call returns true // Find sendMyMail() in /libs/mailer.class.php // Check if the user fills in all fields and the message is sent! if(sendMyMail()) { return true; // Keep true and display onscreen success } }
Even a few words are better than nothing. When you return to edit and work on projects later, it’s often surprising how much you’ll forget. Since you aren’t working with the same variables and function names every day, it’s easy to forget significant portions of your code. Therefore, you can never leave too many comments – but you can leave too many unhelpful comments.
As a general rule, pause and reflect before writing. Ask yourself what is most confusing about the program and how you can best explain it in simple language. Also, consider why you’re writing the code exactly as you are.
Some of the most confusing errors arise when you forget the purpose of custom-built (or third-party) functions. Leave a comment trail leading back to other files if this will help you remember the functionality more easily.
2. Alleviate Some Space!
I can’t emphasize enough the importance of whitespace. This is especially true for PHP and Ruby developers working on massive websites with hundreds of files. You’ll be staring at this code all day long! Wouldn’t it be great if you could easily skim through to the important areas?
$dir1 = "/home/"; // Set main home directory $myCurrentDir = getCurDirr(); // Set the current user directory $userVar = $get_username(); // Current user's username
In the example above, you’ll notice the extra padding I’ve added between the comments and the code on each line. As you scroll through files, this style of commenting will clearly stand out. It makes finding errors and correcting your code significantly easier when variable blocks are clean and organized.
You could apply a similar technique to the code inside a function when you’re confused about how it works. However, this method could clutter your code with inline comments, which is the opposite of orderly! In such cases, I recommend adding a large block comment around the area of logic.
$(document).ready(function() { $('.sub').hide(); // Hide sub-navigation on page load // Check for a click event on an anchor inside .itm div // Prevent the default link action so the page doesn't change on click // Access the parent element of .itm followed by the next .sub list to toggle open/close $('.itm a').live('click', function(e){ e.preventDefault(); $(this).parent().next('.sub').slideToggle('fast'); }); });
This is a small piece of jQuery code targeting a sub-menu sliding navigation. The first comment is inline to explain why we’re hiding all the .sub
classes. Above the live click event handler, I’ve used a block comment and indented all the writing to the same point. This approach makes your comments visually appealing and easier to read – especially for others who may read your code.
3. Comment While Coding
Along with proper spacing, this may be one of the best habits to develop. Nobody wants to go back over their program after it’s working and document every piece. Most of us don’t even want to go back and document the confusing areas! It really does take a lot of effort.
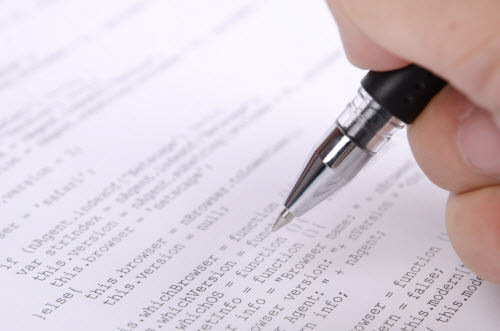
However, if you write the comments while you’re coding, everything will still be fresh in your mind. Developers often get stuck on a problem and search the web for the easiest solution. When you finally solve the problem, there’s usually a moment of clarity when you understand your previous mistakes. This is the perfect time to leave open and honest comments about your code.
Additionally, this practice will help you get used to commenting on all your files. The time required to figure out how something works is much longer after you’ve already built the function. Both your future self and your teammates will appreciate that you left comments ahead of time.
4. Dealing with Buggy Errors
We can’t all sit in front of the computer for hours writing code. While it’s possible to try, at some point, we all need to rest! You may have to part ways with your code for the day, leaving some features still broken. In such cases, it’s crucial to leave long, detailed comments about where you left off.
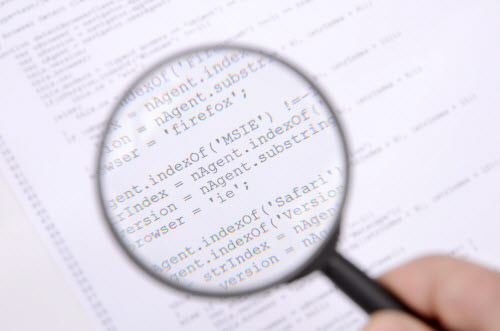
Even after a good night’s sleep, you may be surprised by how difficult it is to get back into the swing of coding. For example, if you’re building an image upload page and have to leave it incomplete, you should comment about where in the process you stopped. Are the images uploading and being stored in temporary memory? Or perhaps they aren’t recognized in the upload form, or they aren’t displayed properly on the page after upload.
Commenting on errors is important for two main reasons. First, you can easily pick up where you left off and try again with a fresh perspective to fix the problem(s). Second, you can differentiate between the live production version of your website and the testing environment. Remember, comments should be used to explain why you’re doing something, not just what it does.
Conclusion
Developing web apps and software is a fulfilling practice, though it can be challenging. If you’re one of the developers who truly understands building software, it’s important to continue maturing your coding skills. Leaving descriptive comments is a good practice in the long run, and you’ll likely never regret it!
If you have suggestions for clearer code commenting, feel free to share them in the discussion area below!