How to Add Keyboard Shortcuts to Your Website
Love keyboard shortcuts? They can help you save a lot of time, right? Would you like to add keyboard shortcuts to your own website, for the benefit of your visitors? It would greatly improve your site’s accessibility and navigation.
In this post, I will give a quick guide on how to add shortcuts to your web page using a JavaScript library called Mousetrap. With Mousetrap you can designate keys like Shift, Ctrl, Alt, Options, and Command to perform particular functions in your website. It also works well across older browsers.
More on Hongkiat:
- Creating animated tooltip easily with Hint.Css
- Building a step-by-step guide using Intro.Js [Tutorial]
- How to style HTML5 range slider
- How to use cookie & HTML5 localstorage
Getting Started
Begin by creating a new HTML document along with the content, and linking the Mousetrap library. I will use the Mousetrap library hosted in CDNjs so that the library will be served through the CloudFlare network, which should be faster than our own server.
<script src="//cdnjs.cloudflare.com/ajax/libs/mousetrap/1.4.6/mousetrap.min.js"></script>
Now we will use the Mousetrap ‘bind’ method to attach keyboard keys to functions. You may assign a single key, a key combination, or sequence keys, for example:
Single Key
In this example, we bind the s.
Mousetrap.bind('s', function(e) { // your function here... });
Combination Key
In this example, we bind the Ctrl and s. You will need to press the two keys together to perform the designated function.
Mousetrap.bind('ctrl+s', function(e) { // your function here... });
Sequence Key
In this example, the user will need to press g and then s subsequently. If you are developing a web-based game, this might be useful for adding a secret hidden key combo.
Mousetrap.bind('g s', function(e) { // your function here... });
Using Mousetrap
We will build a simple web page with a couple of keyboard shortcuts that enable users to access some functionality on the website. We will be using jQuery to make it easier to manipulate the HTML document and select HTML elements.
<script src="//cdnjs.cloudflare.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/mousetrap/1.4.6/mousetrap.min.js"></script>
Let’s begin with something easy.
We are going to bind a key that will allow users to focus on the search input field quickly.
1. The following is the HTML markup for the search along with the id
.
<input type="text" id="search" class="form-control" placeholder="Search for...">
2. Next, we create a function that will focus on the search input.
function search() { var search = $('#search'); search.val(''); search.focus(); }
3. Lastly, we bind a key to run the function.
Mousetrap.bind('/', search);
4. That’s it. You should now be able to navigate to the search input by pressing the / button.
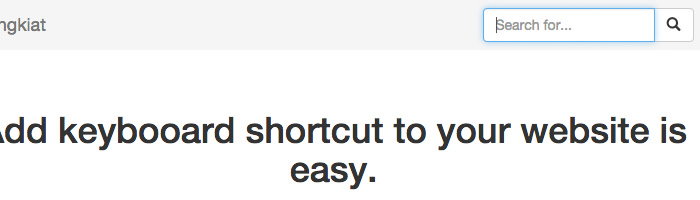
Alternately, you may also bind the key combination, Ctrl/Cmd + F, which is a popular key shortcut used for search in many desktop apps:
Mousetrap.bind(['command+f', 'ctrl+f'], search);
Using Mousetrap with Bootstrap
It is easy to integrate Mousetrap with a framework, for example, Bootstrap. In this second example, we will show a help window that will display a list of the shortcuts available on the website. Here, I opt for the Bootstrap Modal to present the list, and designate the ? key to show the modal.
Although the ? is only accessible with the Shift key, binding just the ? key is sufficient.
Mousetrap.bind('?', function() { $('#help').modal('show'); });
Now when you hit the ? key, a popup will appear.
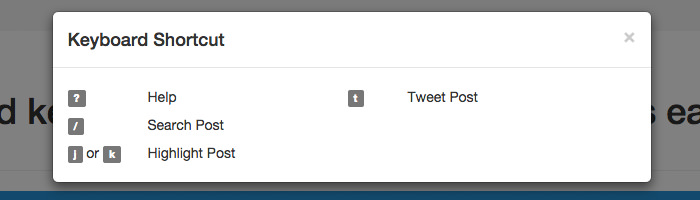
Tip For Efficient Binding
Over time, your growing collection of keyboard shortcuts may start to mess up your code. If this happens, there is an extension you can add to make your ‘key binding’ code more efficient. It is named the ‘bind dictionary’. Add this extension after the primary Mousetrap library, mousetrap.min.js
.
Now, instead of separating each key binding, we can neatly group them in a single bind()
method, like so:
Mousetrap.bind({ '/': search, 't': tweet, '?': function modal() { $('#help').modal('show'); }, 'j': function next() { highLight('j') }, 'k': function prev() { highLight('k') } });
For more advanced implementation, you can see the demo that I’ve made. In the demo, you can press the J or K key to highlight the post, and press T to tweet the current post that you highlighted.