Create an Auto-Login Bot with Python and Selenium
Automation is undoubtedly one of the most coveted skills a programmer can possess. Automation is typically used for tasks that are repetitive, boring, time-consuming, or otherwise inefficient without the use of a script.
With web automation, you can easily create a bot to perform different tasks on the web, for instance to monitor competing hotel rates across the Internet and determine the best price.
Personally, I have always found logging into my email fairly repetitive and boring, so for the sake of a simple example to get you guys started with web automation, let’s implement an automated Python script to log in with a single click to a Gmail account.
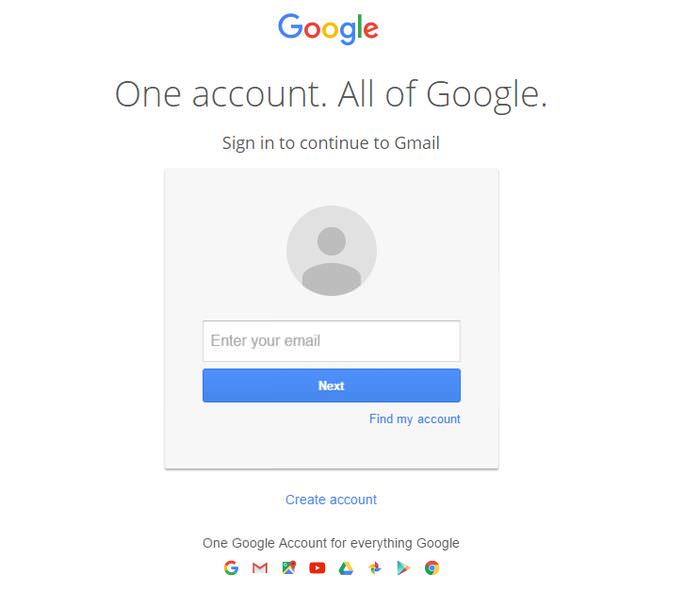
Installation and Setup
In this tutorial we are going to use the following tools:
- Python programming language
- Google Chrome browser
- Selenium browser automation toolkit
- Chrome Driver web driver for Chrome
For our program, we will be using the Python programming language, specifically version 2.7.11. It is critical that we install a fairly new version of Python 2 because it comes with PIP, which will allow us to install third-party packages and frameworks that we will need to automate our scripts.
Once installed, restart your computer for the changes to take effect. Use the command pip install selenium
to add the Selenium web automation toolkit to Python. Selenium will allow us to programmatically scroll, copy text, fill forms and click buttons.
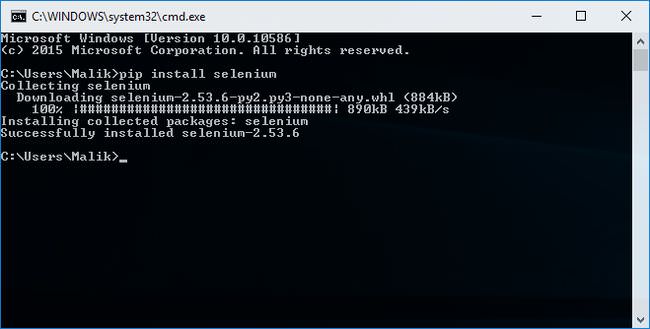
Finally download the Selenium Chrome Driver executable, which will open Google Chrome as needed to perform our automated tasks. The Chrome Driver is simply a way to open Google Chrome (which should already be installed) to access standard browser operations programmatically.
Simply download the most recent ZIP file from here, extract the chromedriver.exe
executable, and place the executable in any directory. Be sure to make note of where your executable is, because we will need it once we get started.
Starting the Program
As aforementioned, we’ll be using the Selenium web automation framework in order to log in programmatically. The first order of business is to import every module we’ll be needing from the Selenium Python library which we installed ealier with PIP.
Let’s open IDLE or another code editor, create a new Python file with .py
extension, and import the following modules:
from selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC
Next, we’ll create two strings that represent our username and password for the email account. Pay attention to capitalization, especially in your password string.
usernameStr = 'putYourUsernameHere' passwordStr = 'putYourPasswordHere'
Now that we have everything set up in order to start the login process, we need to actually instruct a browser window to open Google Chrome, and navigate to Gmail’s login page.
If you haven’t done so already, make sure your Python script is saved in the same location as the chromedriver.exe
executable we extracted earlier.
browser = webdriver.Chrome() browser.get(('https://accounts.google.com/ServiceLogin?' 'service=mail&continue=https://mail.google' '.com/mail/#identifier'))
Finding Our Element
We’ve successfully gotten the browser to open Gmail, but now we need to find the input fields on the web page, into which we can enter our username and password.
We can do this easily using the built-in Chrome Developer Tools. We only need to right-click on the input fields, and select the “Inspect” menu.
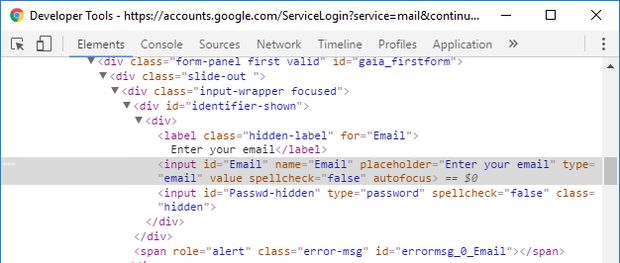
Now it’s just a matter of using HTML id
attributes to find the elements programmatically.
In the code below, we’re simply searching for the username input field by id
, and filling it with the desired text. Then we’re searching for the Next button which transitions with a quick animation before prompting us for the password.
The send_keys()
and click()
commands do exactly as their names suggest — send_keys()
simulates keypresses in the desired element, and click()
simulates a mouse click.
# fill in username and hit the next button username = browser.find_element_by_id('Email') username.send_keys(usernameStr) nextButton = browser.find_element_by_id('next') nextButton.click()
We can do the same thing for the password input field, as well as for the Sign in button. However, these two items appear on the page only after an animated transition.
That said, we need the program to wait a few seconds before resuming its search for elements. For the most part, this stage of code is no different from the previous one. We simply have to instruct the browser to wait a maximum of 10 seconds before locating the password entry.
# wait for transition then continue to fill items password = WebDriverWait(browser, 10).until( EC.presence_of_element_located((By.ID, 'Passwd'))) password.send_keys(passwordStr) signInButton = browser.find_element_by_id('signIn') signInButton.click()
Final Words
You’ve just finished creating a bot that visits a web page, enters your username and password, and successfully sign you in, automating the whole process to a single click. I know this was a simple demonstration, but the possibilities are endless.
On that same note, be careful of how you use this skill. Some people use bots and automated scripts to enter sweepstakes thousands of times, completely disregarding terms and conditions. Others use them for more malicious intentions.
Just be sure to use automation purposefully and carefully, because it really is a much needed skill in the programming community!