How to Create Custom WordPress Template Tags
Building a WordPress Theme is so easy with template tags. You can add the_title()
to display the post or page title, and you can use the_content()
to display post or page contents. There are many more template tags in WordPress that we can use to display other things.
But when it comes to displaying only particular things on your theme, you might want to create your own template tag. In today’s tutorial, we are going to walk you through this not-too-complicated process. Let’s get started with the fundamentals.
Recommended Reading WordPress Conditional Tags (And Snippets) For Beginners
Basic Template Tag
If you take a look at the WordPress Core sources, you will find that a template tag is basically a PHP function running a set of codes with some parameters.
To create your own template tag, you can write a PHP function in functions.php within your theme directory, for example.
function my_template_tag() { echo 'This is my template'; }
Then, in your other theme files, say, single.php
or page.php
, you can add the function, like so.
<?php my_template_tag() ;?>
This will display the ‘This is my template’ that is echoed inside the my_template_tag()
function.
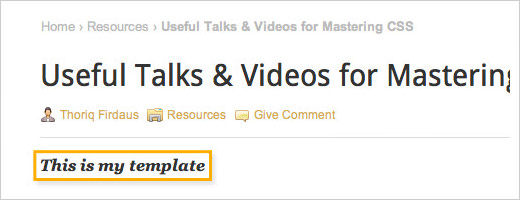
Creating a WordPress Template is really that simple. Alright, let us now take a look at a slightly more advanced example.
Page View Count Template Tag
In this example, we are going to create a template that will show view count for each post and page.
First, install and activate WordPress.com Stat in Jetpack. And make sure that you have connected Jetpack to WordPress.com. The reason we use WordPress.com Stat is that the View data will be stored in WordPress.com rather than in our own database. This could save on our server load.
Create a function in your functions.php named the_view()
, like so:
function the_view() { }
We will put the function (the template tag) in a page or post, so we need to get the page and post ID number. This can be retrieved using get_the_ID();
.
function the_view() { $id = get_the_ID(); }
We can then use a function to retrieve the number of views from WordPress.com Stats by using stats_get_csv();
. This function accepts several parameters ( find the complete list here).
In our case, we need to add the days
, which specify the time range of the view count, and the post_id
.
function the_view() { $id = get_the_ID(); $page_view = stats_get_csv('postviews', 'days=-1&post_id='.$id.''); }
In the code above, since we set the days
parameter to -1
, we will retrieve the view count of the given post ID from the very beginning, from when the WordPress.com Stat plugin is activated.
Once we’ve the number, we just need to echo it, as follows.
function the_view() { $id = get_the_ID(); $page_view = stats_get_csv('postviews', 'days=-1&post_id='.$id.''); echo $page_view[0]['views']; }
That’s it, our new template tag for displaying page view count is done. You can <?php the_view() ;?>
anywhere in page.php or single.php. For example:
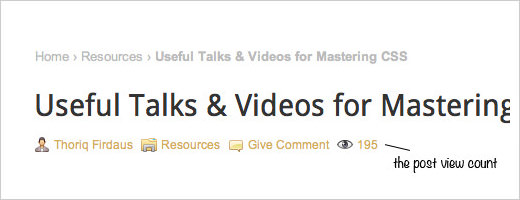
If you have any questions, please feel free to put them in the comment section below.