The Basics of Object-Oriented CSS (OOCSS)
Frontend development moves quickly, with many new techniques added every year. It can be a struggle for developers to keep up with everything. Between Sass and PostCSS it’s easy to get lost in the sea of development tools.
One newer technique is Object-Oriented CSS, also termed OOCSS for short. This isn’t a tool, but rather a CSS writing methodology that aims to make CSS modular and object-based.
In this post, I’d like to introduce the core fundamentals of OOCSS, and how these ideas can be applied to frontend web work. This technique may not catch on with every developer, but it’s worth understanding new concepts to decide if your workflow could benefit from it.
What Makes CSS Object-Oriented?
Object-oriented programming (OOP) is a programming paradigm that focuses on creating reusable objects and establishing relationships between them, as opposed to procedural programming that organizes the code into procedures (routines, subroutines, or functions).
OOP has become widely used in both JavaScript and backend languages in the last few years, but organizing CSS according to its principles is still a new concept.
The “object” in OOCSS refers to an HTML element or anything associated with it (like CSS classes or JavaScript methods). For example, you might have a sidebar widget object that could be replicated for different purposes (newsletter signup, ad blocks, recent posts, etc.). CSS can target these objects en-masse which makes scaling a breeze.
Summarizing OOCSS’ GitHub entry, a CSS object may consist of four things:
- HTML node(s) of the DOM
- CSS declarations about the style of those nodes
- Components like background images
- JavaScript behaviors, listeners, or methods associated with an object
Generally speaking, CSS is object-oriented when it considers classes that are reusable and targetable to multiple page elements.
Many developers would say OOCSS is easier to share with others and easier to pick up after months (or years) of inactive development. This compares with other modular methods like SMACSS, which has stricter rules for categorizing objects in CSS.
The OOCSS FAQ page has a bunch of info if you’re curious to learn more. And the creator Nicole Sullivan often talks about OOCSS and how it ties in with modern web development.
Separate Structure From Style
A big part of OOCSS is writing code that separates page structure (width, height, margins, padding) from appearance (fonts, colors, animations). This allows custom skinning to be applied onto multiple page elements without affecting the structure.
This is also useful for designing components that can be moved around the layout with ease. For example, a “Recent Posts” widget in the sidebar should be moveable into the footer or above the content while maintaining similar styles.
Here’s an example of OOCSS for a “Recent Posts” widget that in this case is our CSS object:
/* Structure */ .side-widget { width: 100%; padding: 10px 5px; } /* Skinning */ .recent-posts { font-family: Helvetica, Arial, sans-serif; color: #2b2b2b; font-size:1.45em; }
Notice that layout is managed with the .side-widget
class that could be applied to multiple sidebar elements as well, while appearance is managed with the .recent-posts
class that could also be used to skin other widgets. For instance, if the .recent-posts
widget were moved to the footer, it might not take the same positioning, but it could have the same look and feel.
Also take a look at this sidebar example from CodePen. It uses a distinct separation of classes for floats and text alignment so that replication will not require extra CSS code.
Separate Container From Content
Separating content from its container element is another important principle of OOCSS.
In simpler terms, this only means that you should avoid using child selectors whenever it’s possible. When customizing any unique page elements like anchor links, headers, blockquotes, or unordered lists, you should give them unique classes rather than descendant selectors.
Here’s a simple example:
/* OOCSS */ .sidebar { /* sidebar contents */ } h2.sidebar-title { /* special h2 element styles */ } /* Non-OOCSS */ .sidebar { /* same sidebar contents */ } .sidebar h2 { /* adds more specificity than needed */ }
Although it’s not horrendous to use the second code format, it’s heavily recommended to follow the first format if you want to write clean OOCSS.
Development Guidelines
It’s tough to nail down exact specifications because developers are constantly debating the purpose of OOCSS. But here are some suggestions that can help you write cleaner OOCSS code:
- Work with classes instead of IDs for styling.
- Try to abstain from multi-level descendant class specificity unless needed.
- Define unique styles with repeatable classes (eg floats, clearfix, unique font stacks).
- Extend elements with targeted classes rather than parent classes.
- Organize your stylesheet into sections, consider adding a table of contents.
Note that developers should still use IDs for JavaScript targeting, but they’re not required for CSS because they’re too specific. If one object uses an ID for CSS styling it can never be replicated since IDs are unique identifiers. If you use only classes for styling then inheritance becomes much easier to predict.
Moreover, classes can be chained together for extra features. A single element could have 10+ classes attached to it. While 10+ classes on one element isn’t something I’d personally recommend, it does allow developers to amass a library of reusable styles for unlimited page elements.
Class names within OOCSS are somewhat controversial, and not set in stone. Many developers prefer to keep classes short and to the point.
Camel case is also popular, for example .errorBox instead of .error-box. If you look at class naming in OOCSS’ documentation you’ll notice camel case is the “official” recommendation. There’s nothing wrong with dashes but as a rule it’s best to follow the OOCSS guidelines.
OOCSS + Sass
Most web developers already love Sass and it has quickly overtaken the frontend community. If you haven’t already tried Sass, it’s worth giving it a shot. It allows you to write code with variables, functions, nesting and compilation methods like mathematical functions.
In competent hands, Sass and OOCSS could be a match made in heaven. You’ll find an excellent writeup about this on The Sass Way blog.
For instance, using the Sass @extend
directive you can apply the properties of one class onto another class. The properties aren’t duplicated but instead, the two classes are combined with a comma selector. This way you can update CSS properties in one location.
If you’re constantly writing stylesheets this would save hours of typing and help automate the OOCSS process.
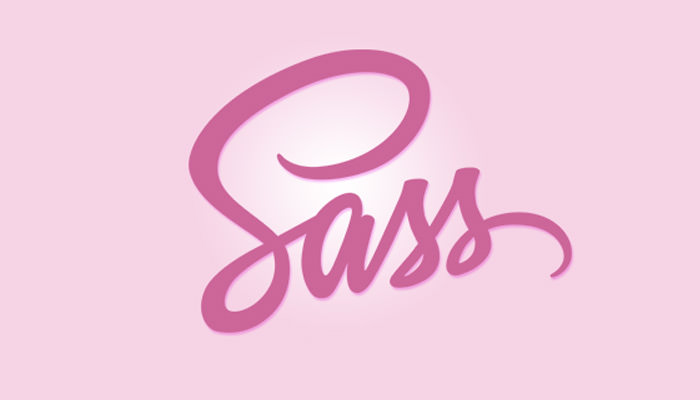
Also remember that code maintenance is a big part of OOCSS. By using Sass, your job gets easier with variables, mixins, and advanced linting tools tied into the workflow.
A key attribute of great OOCSS code is the ability to share it with anyone, even yourself, at a later date and be able to pick it up with ease.
Performance Considerations
OOCSS is meant to operate smoothly and without much confusion. Developers try their best not to repeat themselves at every turn, in fact that’s the premise behind DRY development. Over time, the OOCSS technique can lead to hundreds of CSS classes with individual properties applied dozens of times in a given document.
Since OOCSS is still a new topic, it’s difficult to argue on the topic of bloat. Many CSS files end up bloated with little structure, whereas OOCSS provides rigid structure and (ideally) less bloat. The biggest performance concern would be in the HTML where some elements may accumulate a handful of different classes for layout structure and design.
You’ll find interesting discussions about this topic on sites like Stack Overflow and CSS-Tricks.
My recommendation is to try to build a sample project, and see how it goes. If you fall in love with OOCSS, it may radically change how you code websites. Alternatively, if you hate it you’re still learning a new technique and thinking critically about how it operates. It’s win-win no matter what.
Get Busy Writing OOCSS
The best way to learn anything in web development is to practice. If you already understand the basics of CSS then you’re well on your way!
Since OOCSS doesn’t require preprocessing you could try it with an online IDE, such as CodePen. Simple projects are the best for getting started, and improving your knowledge from there.
Take a look at these resources to further your research in the evolving field of OOCSS.