A Guide To: WordPress Child Themes Development
There are a number of reasons that WordPress developers are starting to use child themes. They give you the opportunity to customize a unique layout on top of another existing theme. This is perfect for beginners who want to play around with building their own themes.
Additionally many premium designs will release new updates over time. If you are making changes to core theme files they will be overwritten when performing an update, but child themes are separate and neatly tucked away. This means you can build off existing premium themes and save loads of time in the process.
In this guide I want to introduce the basic concepts of building a WordPress child theme and why it’s such a good idea.
Recommended Reading: Beginner’s Guide to WordPress Plugin Development
Getting Started
Child themes are not as difficult as they may appear. The benefits of working off a parent theme means you don’t need to write all the HTML/CSS from scratch. A child theme will automatically use any template files you include, such as sidebar.php
or footer.php
. But if they are missing, then your child theme will pull the same files from its parent.
This functionality offers an enormous amount of freedom to customize already existing templates. It’s also great for touching up areas around your website for special events, such as adding design patterns for Christmas or New Years.
Your Required Files
You only need a single .css stylesheet to set up a child theme in WordPress. You also need to create a new directory in the /wp-content/themes
folder which will house your child theme. Pay attention that you aren’t creating this folder inside the parent theme, but right alongside with it in the same themes directory.
Developers will often include a functions.php and screenshot.png in the same folder as your new CSS file. The screenshot is displayed in your WordPress admin panel and the functions theme file can be used for tons of backend changes.
But for now we should focus on the main stylesheet. This is commonly named style.css and includes a comment header with key meta information. This is important because your theme will only display as a child if you include the parent’s directory name. Below is an example header comment:
/* Theme Name: Twenty Eleven Child Theme URI: http: //example.com/ Description: Child theme for the Twenty Eleven design Author: Jake Rocheleau Author URI: http: //www.hongkiat.com/blog/ Template: twentyeleven Version: 0.1 */
The value for template should be the directory name for the accompanied parent theme. Other than that all the other tags should be familiar to standard WordPress theming.
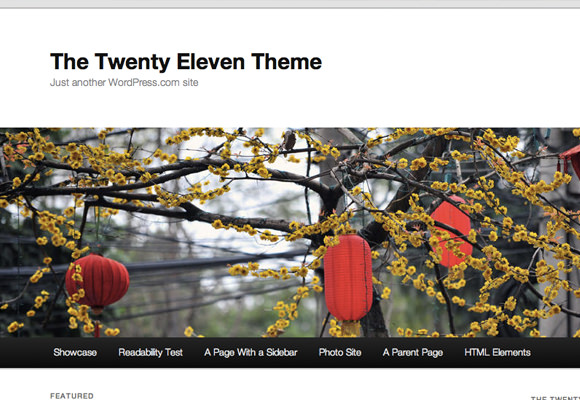
Although all the parent PHP templates will be used, the original parent’s style.css will not be imported automatically. If you want to work off the original styles you’ll have to include it at the top of your child’s style.css document. Below is an example including the WP Twenty Eleven theme.
@import url("../twentyeleven/style.css");
Setting Up New Styles
Applying CSS rules to your theme is just as easy as editing the original. If you know which elements you need to target then use the same selectors in your own child theme.
We could demo with some really easy changes to links and paragraphs. I’ve used code from the original Twenty Eleven theme for targeting the different elements. At times it is necessary to use a more specific selector to override the older design.
body { padding: 0 1.4em; } #page { margin: 1.667em auto; max-width: 900px; } a { color: #5281df; text-decoration: none; font-family: Calibri, Tahoma, Arial, sans-serif; } a:focus, a:active, a:hover { text-decoration: underline; }
In these changes I’ve reduced the overall body size and also removed some padding from the edges. All of these selectors can be found listed in the original .css document. It’s notable that I’m also changing some properties for all anchor links which include a different font stack and color choice.
The !important Things
CSS has a special declaration to mark priority above other styles. The syntax is displayed as !important
beginning with the exclamation mark and terminating at the end of your CSS property. This is necessary if you have cascading styles from a parent theme which are overriding your own custom rules.
a { color: #5281df !important; text-decoration: none; font-family: Calibri, Tahoma, Arial, sans-serif; }
Above I have copied my original changes and edited the anchor text color with an important clause. This will take precedence over all other styles of the same selector depth. More defined elements (such as #access li:hover > a
) will usually hold their own styles unless the color
was still inherited from our original selector. In this case our parent theme does not setup a font-family property on anchor links, so as such we don’t run into any inheritance issues.
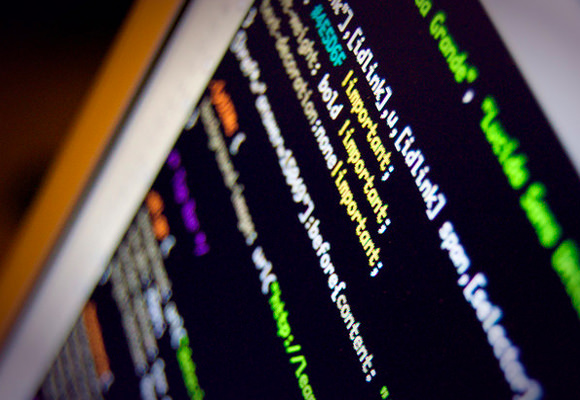
If you are having trouble making your changes stick, try popping one of these important marks at the end of your property statement. This isn’t a perfect fix for every inheritance problem, but it does come in handy a lot more frequently than you’d think.
Cloning functions.php
Unlike the main stylesheet, your child theme will import its parent’s functions automatically. This means you don’t need to copy over any of the PHP code to still have it active in your new theme. Yet if you’d like to re-define some of the functions you can build another functions.php and write in your new code with any changes.
As an example I have built a function which parses a few JavaScript files when the template initiates. This will remove any older versions of jQuery and SWFObject scripts, while simultaneously adding the most recent versions to the wp_head
area.
// queue js files for load function mytheme_js() { if (is_admin()) return; wp_deregister_script('jquery'); wp_register_script('jquery', 'http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js'); wp_enqueue_script('jquery'); wp_deregister_script('swfobject'); wp_register_script('swfobject', 'http://ajax.googleapis.com/ajax/libs/swfobject/2.2/swfobject.js'); wp_enqueue_script('swfobject'); } add_action('init', mytheme_js);
I should point out that if you are importing code from the parent functions.php then you’ll have to use a different function name. Otherwise PHP will give out a fatal error and you will have to FTP into the server to fix the mistake.
Working with Theme Files
The most broad category of theming is building custom layouts and page types. By default your child theme will inherit all of its parent’s theme files. But you have the option of creating new child theme files and WP will register these as the ‘primary’ template.
For example archive.php and index.php are used to display the post archives and homepage screen, respectively. If there are changes you’d like to make which require edits to the HTML then you’d be safer cloning the parent files and editing them in the child’s theme directory.
Custom Page Templates
While we’re talking about template files I also want to introduce a piece of WordPress functionality which many are not familiar with. You can build page and post templates which will be selectable from the Admin panel when creating new content. Even if the parent theme doesn’t have the new custom template file WordPress will still use the child in place of a page.php or single.php.
First create a new file named page-offer.php. This will be a “special offer” promotional page which is themed differently than all the others. In here you can copy over your original page code or even build the theme entirely from scratch. The only code we need to let WordPress know about this new template is a comment setup in PHP.
<?php /** * Template Name: Offer */ ?>
Another alternative to this method is building custom pages named after the unique ID number. So instead of loading the default archive.php for author pages you could create a file such as author-ID.php where ID is the user’s unique WordPress ID number. Although this system is more taxing since you’d need to create a new template file for each of the authors on your site.
It becomes more useful if you can combine these two techniques with other template files. Notably categories and tags work well using their own theme files. Also if you link to attachments in your content then you’ll want to consider the different possible template layouts for each mime type. I included the template hierarchy below for a simple JPEG image attachment:
- image.php
- jpeg.php
- image_jpeg.php
- attachment.php
Additional Resources
Along with all the tips in this guide I want to share a set of important links for theme developers. There are already so many great articles and free child themes you can check out to study deeper into this subject. I added a wonderful collection of these resources below:
- How to build a WordPress Child Theme using Hooks and Filters
- A Few Words on Child Themes
- How to Create, Modify, and Use Child Themes in WordPress
Conclusion
I hope the process of building WordPress child themes is clearer for you after reading this article. I’ve tried to explain how child themes can inherit both CSS and PHP templates from a parent. Additionally it’s very simple to manipulate specific files and create your own unique themes.
The WordPress 3.0 release featured a lot more integrated support for child themes. It’s such a powerful time to move into WP development. Try out these tricks on your own site and see if you can build a complementary child theme. Also if you’ve built any great child themes in the past we would love to check them out. Let us know your thoughts and suggestions in the post comments area.