LESS CSS – Beginner’s Guide
CSS Pre-processors have now become a staple in web development. They enhance plain CSS with programming features such as Variables, Functions or Mixins, and Operations, allowing web developers to construct modular, scalable, and more manageable CSS styles.
In this post, we are going to explore LESS, which is one of the most popular CSS Pre-processors available. It has been widely implemented in numerous front-end frameworks, including Bootstrap. We will also guide you through the basic utilities, tools, and setups to help you get started with LESS.
The Compiler
Let’s start by setting up a compiler. The syntax of LESS is non-standard according to the W3C specification. This means that browsers cannot process and render the output, even though LESS shares similar traits with CSS.
Here’s a quick look at some LESS code:
@color-base: #2d5e8b; .class1 { background-color: @color-base; .class2 { background-color: #fff; color: @color-base; } }
The compiler will process the code and turn LESS syntax into browser-compliant CSS format:
.class1 { background-color: #2d5e8b; } .class1 .class2 { background-color: #fff; color: #2d5e8b; }
There are a number of tools for compiling CSS:
Using JavaScript
LESS comes with a less.js
file which is really easy to deploy in your website. Create a stylesheet with .less
extension and link it in your document using the rel="stylesheet/less"
attribute.
<link rel="stylesheet/less" type="text/css" href="main.less" />
You can obtain the JS file here, download it through Bower package manager, else directly link to CDN, like so:
<link rel="stylesheet/less" type="text/css" href="main.less" /> <script src="//cdnjs.cloudflare.com/ajax/libs/less.js/2.5.1/less.min.js"></script>
You are all set and can compose styles within the .less
. The LESS syntax will be compiled on the fly as the page loads. Keep in mind that using JavaScript is discouraged at the production stage as it will badly affect the website performance.
You should always compile the LESS syntax beforehand and only serve regular CSS instead. You can use Terminal, a Task Runner like Grunt or Gulp, or a graphical application to do so.
Using CLI
LESS provides a native command line interface (CLI), lessc
, which handles several tasks beyond just compiling the LESS syntax. Using the CLI we can lint the codes, compress the files, and create a source map. The command is based on Node.js that effectively allows the command to work across Windows, OS X, and Linux.
Make sure that Node.js has been installed (otherwise grab the installer here), then install LESS CLI through NPM (Node Package Manager) using the following command line.
npm install -g less
Now you have the lessc
command at your disposal to compile LESS into CSS:
lessc style.less style.css
Using Task Runner
Task runner is a tool that automates development tasks and workflows. Instead of manually running the ‘lessc’ command each time we want to compile our code, we can install a task runner. This tool can be set to monitor changes within our LESS files and instantly compile LESS into CSS.
Two popular tools in this category are Grunt and Gulp. We have a series of posts that cover these tools. Check out these posts to learn how to integrate these tools into your workflow.
- How to Automate Your Workflow using Grunt
- Getting Started With Gulp.js
- The Battle Of Build Scripts: Gulp Vs Grunt
Using a Graphical Application
For those who are not familiar with using the Terminal and command lines, a graphical interface is a viable alternative. Today, there are numerous applications available for compiling LESS across all platforms, some of which are free, while others require payment.
Here is the complete list:
App | Platform | Cost |
Mixture | Mac / Windows | Free |
Koala | Mac / Windows / Linux | Free |
Prepros | Mac / Windows | Freemium ($29) |
WinLESS | Windows | Free |
CodeKit | Mac | $32 |
The choice of compiler (beyond JavaScript) doesn’t significantly impact your work, as long as the tool functions effectively and enhances your workflow. Feel free to choose the one that suits you best.
The Code Editor
You have the flexibility to use any code editor of your choice. All you need to do is install a plugin or an extension that highlights LESS syntax with appropriate colors. This feature is now widely available across almost all code editors and Integrated Development Environments (IDEs). Some of the popular ones that support this feature include Sublime Text, Notepad++, Visual Studio, and TextMate, among others.
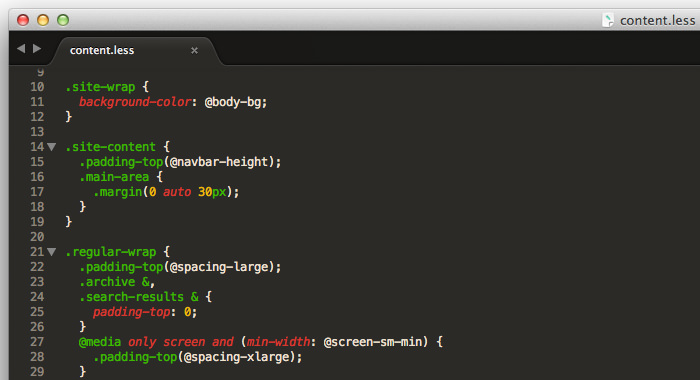
Now that we have the compiler and the code editor all set, we can start writing CSS styles with LESS syntax.
LESS Syntax
Unlike regular CSS as we know it, LESS works much more like a programming language. It’s dynamic, so expect to find some terminologies like Variables, Operation and Scope along the way.
Variables
First of all, let’s take a look at the Variables.
If you’ve been working quite awhile with CSS, you probably have written something like this, where we have repetitive values assigned in declaration blocks in the entire stylesheet.
.class1 { background-color: #2d5e8b; } .class2 { background-color: #fff; color: #2d5e8b; } .class3 { border: 1px solid #2d5e8b; }
This practice is actually fine – until we find ourselves having to sift through more than a thousand or more similar snippets throughout the stylesheet. This could happen when building a large-scale website. Work will become tedious.
If we are using a CSS pre-procesor like LESS, the instance above would not be a problem – we can use Variables. The variables will allow us to store a constant value that later can be reused in the entire stylesheet.
@color-base: #2d5e8b; .class1 { background-color: @color-base; } .class2 { background-color: #fff; color: @color-base; } .class3 { border: 1px solid @color-base; }
In the example above, we store the color #2d5e8b
in the @color-base
variable. When you want to change the color, we only need to change the value in this variable.
Aside from color, you can also put other values in the variables like for example:
@font-family: Georgia @dot-border: dotted @transition: linear @opacity: 0.5
Mixins
In LESS, we can use Mixins to reuse whole declarations in a CSS rule set in another rule set. Here is an example:
.gradients { background: #eaeaea; background: linear-gradient(top, #eaeaea, #cccccc); background: -o-linear-gradient(top, #eaeaea, #cccccc); background: -ms-linear-gradient(top, #eaeaea, #cccccc); background: -moz-linear-gradient(top, #eaeaea, #cccccc); background: -webkit-linear-gradient(top, #eaeaea, #cccccc); }
In the above snippet, we have preset a default gradient color inside the .gradients
class. Whenever we want to add the gradients we simply insert the .gradients
this way:
div { .gradients; border: 1px solid #555; border-radius: 3px; }
The .box
will inherit all the declaration block inside the .gradients
. So, the CSS rule above is equal to the following plain CSS:
div { background: #eaeaea; background: linear-gradient(top, #eaeaea, #cccccc); background: -o-linear-gradient(top, #eaeaea, #cccccc); background: -ms-linear-gradient(top, #eaeaea, #cccccc); background: -moz-linear-gradient(top, #eaeaea, #cccccc); background: -webkit-linear-gradient(top, #eaeaea, #cccccc); border: 1px solid #555; border-radius: 3px; }
Furthermore, if you are using CSS3 a lot in your website, you can use LESS Elements to make your job much easier. LESS Elements is a collection of common CSS3 Mixins that we may use often in stylesheets, such as border-radius
, gradients
, drop-shadow
and so on.
To use LESS Elements, simply add the @import
rule in your LESS stylesheet, but don’t forget to download it first and add it into your working directory.
@import "elements.less";
We can now reuse all the classes provided from the elements.less
, for example, to add 3px
border radius to a div
, we can write:
div { .rounded(3px); }
For further usage, please refer to the official documentation.
Nested Rules
When you write styles in plain CSS, you may also have come through these typical code structures.
nav { height: 40px; width: 100%; background: #455868; border-bottom: 2px solid #283744; } nav li { width: 600px; height: 40px; } nav li a { color: #fff; line-height: 40px; text-shadow: 1px 1px 0px #283744; }
In plain CSS, we select child elements by first targeting the parent in every rule set, which is considerably redundant if we follow the “best practices” principle.
In LESS CSS, we can simplify the rule sets by nesting the child elements inside the parents, as follows;
nav { height: 40px; width: 100%; background: #455868; border-bottom: 2px solid #283744; li { width: 600px; height: 40px; a { color: #fff; line-height: 40px; text-shadow: 1px 1px 0px #283744; } } }
You can also assign pseudo-classes, like :hover
, to the selector using the ampersand (&) symbol.
Let’s say we want to add :hover
to the anchor tag above, we can write it this way:
a { color: #fff; line-height: 40px; text-shadow: 1px 1px 0px #283744; &:hover { background-color: #000; color: #fff; } }
Operation
We can also perform operations in LESS, such as addition, subtraction, multiplication and division to numbers, colors and variables in the stylesheet.
Let’s say we want element B to be two times higher than element A. In that case, we can write it this way:
@height: 100px .element-A { height: @height; } .element-B { height: @height * 2; }
As you can see above, we first store the value in the @height
variable, then assign the value to element A.
In element B, rather than calculate the height ourselves, we can multiply the height by 2 using the asterisk operator (*). Now, whenever we change the value in the @height
variable, element B will always have twice the height.
Check out more advanced operation examples in our previous tutorial: Designing A Slick Menu Navigation Bar.
Scope
LESS applies the Scope concept, where variables will be inherited first from the local scope, and when it is not available locally, it will search through a wider scope.
header { @color: black; background-color: @color; nav { @color: blue; background-color: @color; a { color: @color; } } }
In the example above, the header
has a black background color, but nav
‘s background color will be blue as it has the @color variable in its local scope, while the a
will also have blue that is inherited from its nearer parent, nav
.
Final Thoughts
Ultimately, we hope this post has provided you with a fundamental understanding of how to improve your CSS writing using LESS. It may feel a bit unfamiliar at first, but with more practice, it will undoubtedly become much easier.
Here are a couple of tutorials that I encourage you to look into for further tips and practices, which can help push your LESS skill up to the next level.