How to Use WordPress Action Hooks in Theme Customization
WordPress child themes give a relatively easy way to customize the look and feel of a theme. If the theme’s options don’t provide you with adequate design choices, you can just add a new rule to the child theme’s default stylesheet file called style.css. But what happens when you also want to modify the theme’s functionality? That is one of the cases when WordPress actions come to your help.
WordPress has become so popular partly because of its high customizability. The WordPress Core is loaded with different hooks that enable developers to modify or enhance the default functionality. Moreover, we are allowed to include custom hooks in our themes and plugins to help other developers to easily adjust our code to their needs.
About WordPress Hooks
WordPress hooks work somewhat similar to real-life hooks in the sense that you can catch the fish you want at the right spot if you properly use them.
You can remove a caught function (e.g. you can remove the WordPress admin bar for low-level users), you can leave it intact and enhance it with your own functionality (e.g. you can add more menus or widget areas to a theme), or you can override it (e.g. you can modify the behaviour of a core function).
There are two different kind of hooks in WordPress: actions and filters. In this post we will take a look at how we can make use of action hooks in theme customization.
How WordPress Hooks Work
To use a very simple language, actions indicate that something has happened during the WordPress page lifecycle: certain parts of the site have been loaded, certain options or settings have been set up, plugins or widgets have been initialized, and so on.
Filters are different from actions in their nature. They are used to pass data through, and modify, manage or intercept it before rendering it to the screen or saving user data into the database.
At every significant landmark of the WordPress page lifecycle there is either an action or a filter hook to which we can add our custom code to modify the default behaviour to our needs.
The certain actions and filters running during a request depend on which page was requested by the user agent: for example in a single post request hooks related to single posts are available, but hooks related to other parts of the site (e.g. the admin area) aren’t.
Find Action Hooks
The Action Reference of the WordPress Codex gives a detailed overview of the actions running through different requests. The important thing is that if we want to accomplish a task we need to hook into the right place, not before or after it, otherwise the action won’t be completed.
So for example if we want to add our Google Analytics code to a site we need to hook our action right before the footer is loaded.
If we speak about theme customization, action hooks can come from two different places: from WordPress Core and the theme itself. There are themes that don’t have hooks at all, but others provide developers with some or many – it’s always the theme author’s choice. The default Twenty Fifteen Theme has only one action hook for footer customization under the name of ‘twentyfifteen_credits’.
If you like to browse source code, you can also find action hooks easily. Action hooks are added to the code with the do_action() WordPress function.
If you run a quick search for the expression ‘do_action’ in a more advanced code editor – like I did in Eclipse below – you can see a list about the spots where you can hook your custom functionality into the core. I searched in the /wp-includes/ folder, but you can also run a search for the /wp-admin/ folder that contains the action hooks related to the WordPress dashboard (admin area).
The good thing is that the names of the action hooks are usually pretty self-explanatory, but there is usually a nice comment inside the code that can give you more knowledge whether the given action hook is good for the reason you want to use it for.
For example the code comment before the ‘widgets_init’ action hook says that it “fires after all default WordPress widgets have been registered”. If you take a peek at the code before this action hook, you can find all the default WP widgets’ initialization before it – so you can be sure that the comment didn’t lie, and if you want to register your own custom widget, this will be the right spot.
In many cases the source code provides us with much more information than the Codex, so it can be a good idea to learn how to quickly navigate in it.
Add Your Own Actions
When you want to add your own action, you need to create a custom function and bind this function to a specific action hook by using the add_action() WordPress function. Custom actions added with the add_action() function are usually triggered on the spot when the core calls the appropriate do_action() function.
Let’s see a simple example.
How To Find The Action Hook You Need
Let’s say you want to add your custom favicon to your site. First, you need to find the right action hook you can bind your own functionality to.
Let’s think. If you wanted to add a favicon to a plain HTML page where would you put it? Of course, you need to place it inside the <head> section of the HTML file with the following markup:
<link rel="shortcut icon" href="/https://assets.hongkiat.com/uploads/wp-action-hooks-theme-customization/your-favicon.ico" type="image/x-icon" />
So the action hook you need must be related to the loading of the <head>
section.
(1) Open the Action Reference, and see what it has to offer. We are lucky, as if we browse through the actions, we can only find one, wp_head, that based on its name has the possiblity to be related to the loading of the <head> section.
(2) To be sure, let’s check the documentation in the WordPress Codex. The Codex advises that “you use this hook by having your function echo output to the browser”, so right now it seems to be perfect for us. But let’s check it in the source code.
(3) As this hook is not related to the admin area we will need to run our search in the /wp-includes/ folder. If we search for the word ‘wp-head’ we will get many results as this specific action is used by WP Core many times.
We need to look for the spot where it gets defined, so search for the expression do_action( ‘wp_head’. Note that we didn’t finish the parentheses, as we can’t be sure yet if this action has parameters or not.
Eclipse returns only one result that can be found inside the /wp-includes/general-template.php file. The comment before the action hook definition says that it “prints scripts or data in the head tag on front end”, so now we can be dead-sure that wp_head is the action hook we need.
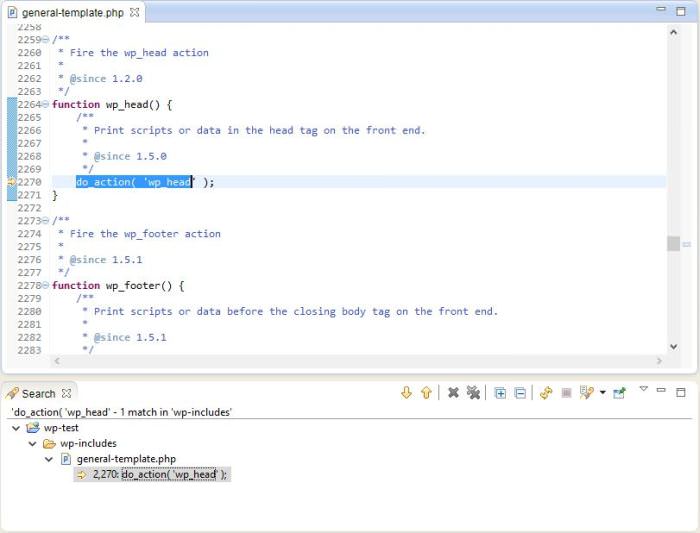
Checking For Parameters
When you add your own actions you also need to be sure if the hook you want to use takes parameters or not. You can easily find this out by looking at the do_action() function.
The syntax of the do_action() function is the following:
do_action( 'name_of_action'[, $parameter1, $parameter2, ...] )
Only the name of the action is required, the parameters are optional. If you find arguments in the relevant call of the do_action() function, you need to include them in the declaration of the custom function you create.
If you don’t find any, then your custom function must work without arguments. In the do_action() definition of the wp_head action hook, there are no parameters.
Let’s compare it to an action hook that takes a parameter. The action hook called ‘wp_register_sidebar_widget’ takes one parameter that you always have to pass to the custom function you bind to the hook.
Let’s see the difference in the do_action() syntax of the two cases:
do_action( 'wp_head' ); do_action( 'wp_register_sidebar_widget', $widget );
In the first case there’s no parameter, so the custom function will use the following syntax:
function my_function_without_parameters() { ... }
In the second case there is one parameter that you always have to pass as an argument into the declaration of your custom function:
function my_function_with_parameters( $widget ) { ... }
How To Hook Your Custom Function In
Now we know everything we need. Let’s create our custom function that will display a favicon on our site.
First, create a new function without any arguments, then bind it to the wp_head action hook with the help of the add_action() WordPress function.
function custom_add_favicon() { echo '<link rel="shortcut icon" href="/https://assets.hongkiat.com/uploads/wp-action-hooks-theme-customization/your-favicon.ico" type="image/x-icon" />'; } add_action( 'wp_head', 'custom_add_favicon');
You need to pass the name of the action hook to the add_action() function as an argument first, then you need to add the name of your custom function.
These are the two required parameters of add_action(). It has two optional parameters too, priority and accepted arguments. Let’s see how to use these.
Define Priorities
It happens in many cases that there are more than one action bound to the same hook. So which one will be executed first? This is where we can use the $priority optional parameter of the add_action() function.
We add the priority as a positive integer, the default value being 10. If we want an action to be executed early, we give it a lower value, if we want it to be executed later, we give it a higher value.
So if we think that the favicon needs to be there early, we can enhance our previous add_action() call in the following way:
add_action( 'wp_head', 'custom_add_favicon', 5);
Please note that the priorities always have to be set relatively to the other custom functions that use the same action hook.
Add The Number of Accepted Arguments
You are required to add the number of accepted arguments in case you use an action hook that takes parameters. Let’s see the example we used before.
The action hook ‘wp_register_sidebar_widget’ takes one parameter, so when we bind our custom function to this hook, we also need to include this as an argument when we call the add_action() function.
Our code in this case will look like this:
function my_sidebar_widget_function( $widget ) { // Your code } add_action( 'wp_register_sidebar_widget', 'my_sidebar_widget_function', 10, 1);
Note that we must also add the priority (we chose the default 10 here) to make sure that WordPress knows what each parameter means. If we omitted the priority, WordPress could suppose that 1 is the priority which is not true, as it indicates the number of the accepted arguments.
Conclusion
You can make many experiments with action hooks in theme customization. For example you can add your custom scripts (JS) and styles (CSS) with the wp_enqueue_scripts action hook, or your Google Analytics code with the wp_footer action hook.
You not only can add your own actions, but you can also remove complete functionalities from the WordPress core with the use of the remove_action() function that uses the same logic as add_action().
If you are a theme author, and you want to make an extensible theme, it can be a good idea to add your own custom action hooks to the appropriate template files with the do_action() function.
If you want to do so, think carefully about parameters that other developers who will use your theme will have to pass as arguments when they want to hook in their custom functionalities.
While designing the locations of your theme’s custom action hooks don’t forget that it does not make much sense to include custom theme hooks on the same spots where the WordPress Core itself has its own hooks.