10 WordPress Template Tags You May Not Know
WordPress is shipped with quite an abundance of Template Tags since its inception. These Template Tags in WordPress are PHP functions that can be used to output as well as retrieve a piece of data.
If you have been developing a WordPress theme, you may be familiar with some of these Template Tags, such as the_title
that shows the post title, the_author
that shows the name of the post’s author, and the link of the post.
WordPress keeps evolving. Every new release often introduces a few new Template Tags. So much so that keeping up with all these Template Tags — both old and new — can be quite challenging. Check out these 20 template tags you might have overlooked.
Read Also: How to Create Custom WordPress Template Tags
Capital P
WordPress, as per their guideline and standard, has to be written with the capital P i.e. WordPress is a no-no; the right way is to spell it as WordPress.
The capital “P” is an issue with so much importance to the extend that Matt Mullenweg (founder of WordPress) has included it in his resolution back in 2009. The capital_p_dangit()
function is introduced as part of the initiative.
Since: 3.0.0
// Using it straightforwardly $footer_text = get_theme_mod( "footer_text", "" ); $footer_text = captial_p_dangit( $footer_text ); // Any WordPress text is turned with capital P. // Or, using it in a WordPress Filter. add_filter( "the_excerpt", function( $text ) { return captial_p_dangit( $text ); } );
Custom Logo
In 4.5, WordPress introduced the ability to upload a logo for themes through the Customizer. This new feature requires theme support: by adding add_theme_support( 'site-logo' )
, the logo will appear in the Customizer.
This feature leads to the use of a few new Template Tags that can handle the logo image output on Themes, namely: has_custom_logo()
, get_custom_logo()
, and the_custom_logo()
.
Since: 4.5.0
// 1. Output includes the image logo and the link back to home. the_custom_logo(); // 2. Get the custom logo output "string". $logo = get_custom_logo(); // 3. Conditional if ( has_custom_logo() ) { $logo = get_custom_logo(); } // 4. Using the 'get_custom_logo' to wrap the logo with a div; add_filter( "get_custom_logo", function( $html ) { return ''. $html .''; } );
Thumbnail Image URL
WordPress has a long-integrated, native utility to add a thumbnail image or featured image. The Template Tag, the_post_thumbnail()
, shows the image tag along with their attributes.
But what if you want to show the image thumbnail as a background through CSS instead? Use the Template Tag, get_the_post_thumbnail_url()
.
Since: 4.4.0
Example:
<?php echo get_the_post_thumbnail_url(); // e.g. "http://example.com/path/to/image/thumbnail.jpg"?> <div class="image-thumbnail" style="background-image; url(<?php echo get_the_post_thumbnail_url() ?>)"></div>
Generate Random Number
This Template Tag will give you a random number based on a specified range. WordPress is using this function internally to generate a random password. You can probably use it to generate a random coupon number for your WooCommerce site.
Since: 2.6.2
Example:
// Generate a number from 1 to 200 $rand_number = wp_rand( 1, 200 ); // output will not be below 0 or 201 above.
Comments Pagination
Most Themes are currently using the the_comments_navigation()
which will give the “Next” and “Prev” type of navigation link. If you want to show a numbered navigation (pagination), replace the tag with the_comments_pagination()
instead.
Bear in mind that the Template Tag is only available in WordPress 4.4.0 upwards. Make sure to run a check before deploying it.
Since: 4.4.0
Example:
<?php // Replace the `the_comments_navigation()` if ( function_exists( 'the_comments_pagination' ) ) { the_comments_pagination(); } else { the_comments_navigation(); } <ol class="comment-list"> <?php wp_list_comments( array( 'style' => 'ol', 'short_ping' => true, 'avatar_size' => 42, ) ); ?> </ol> <!-- .comment-list -->
Shortening URL
This Template Tag will shorten a url length. And such a very long URL won’t break into a new line within the body content. There are 2 options you can take: add overflow-wrap: break-word;
in your CSS, or trim the length of the URL with the url_shorten()
Template Tag.
Since: 1.2.0
Example:
$link = get_the_permalink(); $url_text = url_shorten( $link ); // e.g. www.hongkiat.com/blog/css... echo '<a href="'. $link .'">'. $url_text .'</a>';
Add Inline Scripts
We have always used the wp_enqueue_script
to register, load a script and its dependencies. Loading an internal script however was not quite straightforward, until this Template Tag, wp_add_inline_script
is introduced.
Adding an inline script requires a known enqueued script to which it will be attached. This handler is passed as the first parameter of the script similar to the wp_localize_script()
function. The second parameter should pass the content of the script. The third parameters specifiy whether the inline should be output ‘before’ or ‘after’ .
Since: 4.5.0
Example:
function enqueue_script() { wp_enqueue_script( 'twentysixteen-script', get_template_directory_uri() . '/js/functions.js', array( 'jquery' ), '20160412', true ); wp_add_inline_script( 'twentysixteen-script', 'window.hkdc = {}', 'before' ); } add_action( 'wp_enqueue_scripts', 'enqueue_script' ); // Output: // <script type='text/javascript'>window.hkdc = {}</script> // <script type='text/javascript' src='http://local.wordpress.dev/wp-content/themes/twentysixteen/js/functions.js?ver=20160412'></script>
Dropdown Language
The wp_dropdown_languages
Template Tag will output an HTML option showing a list of languages in your WordPress site. You will find this template tag useful if you need to localize your website. You can use it to show your language options in the User Editor screen or in the front-end of your site to allow users to select their language preference.
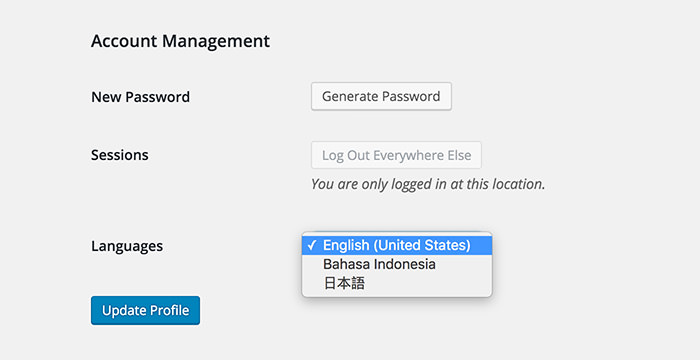
Since: 4.0.0
Example:
wp_dropdown_languages( array( 'id' => 'lang_options', 'name' => 'lang_options', 'languages' => get_available_languages(), 'translations' => array( 'id_ID', 'ja' ), // Indonesia, and Japan 'selected' => 'en_US', 'show_available_translations' => false, ) );
Get the Avatar Image URL
As the name suggests, this Template Tag, get_avatar_url()
, will retrieve the image path of the user’s avatar. It allows you to display and mold the avatar in any way you like, instead of simply displaying it through the HTML image tag.
Since: 4.2.0
Example:
$avatar = get_avatar_url( 'admin@domain.com' ); <div class="avatar-url" style="background-image: url(<?php echo $avatar; ?>)"> </div>
Get Theme
This function retrieves an object containing information of the currently active Theme. This information includes the theme Slug, Name, Version, Text Domain, Author, etc.
In the following code snippet, we use it to retrieve the version and pass it as the script version.
Since: 3.4.0
Example:
$theme = wp_get_theme(); define( 'THEME_SLUG', $theme->template ); //twentysixteen define( 'THEME_NAME', $theme->get( 'Name' ) ); // Twenty Sixteen define( 'THEME_VERSION', $theme->get( 'Version' ) ); //1.2 function load_scripts() { wp_enqueue_script( 'script-ie', $templateuri .'js/ie.js', array( "jquery" ), THEME_VERSION ); wp_script_add_data( 'script-ie', 'conditional', 'lt IE 9' ); } add_action( 'wp_enqueue_scripts', 'load_scripts' );