10 Coolest Features in jQuery 3
jQuery 3.0, the new major release of jQuery finally got released. The web developer community waited for this important step since October 2014, when the jQuery team began to work on the new major version up until now, June 2016, when the final release is out.
The release note promises a slimmer and faster jQuery, with backwards compatibility in mind. In this post, we have a look at some of the new features of jQuery 3.0 to give you an overview about how it changes the JavaScript landscape.
Where to Start
If you want to download jQuery 3.0 to experiment for yourself, go right to the download page. It’s also worth having a look at the Upgrade Guide, or the source code.
If you want to test how your existing project works with jQuery 3.0, you can give a try to the jQuery Migrate plugin that identifies compatibility issues in your code. You can also take a peek into future milestones.
This article doesn’t cover all the new features of jQuery 3.0, only the more interesting ones: better code quality, integration of new ECMAScript 6 features, a new API for animations, a new method for escaping strings, increased SVG support, improved async callbacks, better compatibility with responsive sites, and increased security.
1. Old IE Workarounds Got Removed
One of the main goals of the new major release was to make it faster and sleeker, therefore the old hacks and workarounds related to IE9- got removed. This means if you want or need to support IE6-8, you’ll have to keep using the latest 1.12
release, as even the 2.x
series doesn’t have full support for older Internet Explorers (IE9-). Check out the notes on browser support in the docs.
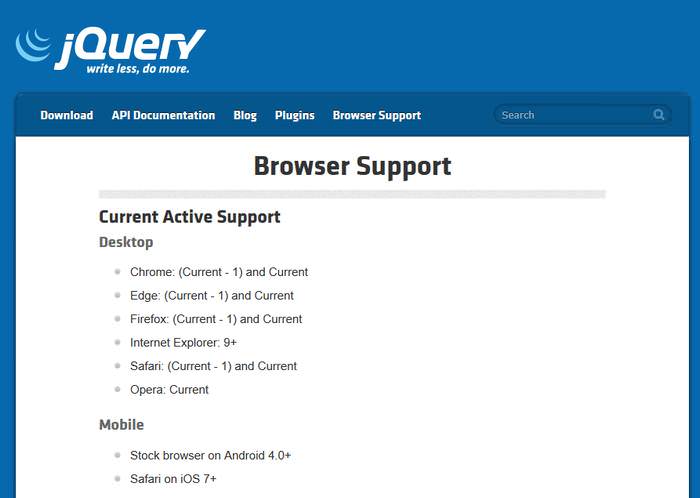
Note that there are also many deprecated features in jQuery 3. A big shortcoming of the Upgrade Guide is that the deprecated features – as of June 2016 – aren’t grouped, so if you’re interested in them, you’ll need to look them up with your browser’s search tool (Ctrl+F).
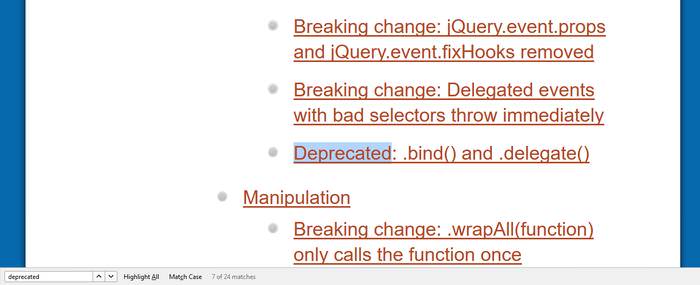
2. jQuery 3.0 Runs in Strict Mode
As most browsers supported by jQuery 3 support strict mode, the new major release have been built with this directive in mind.
Although jQuery 3 has been written in strict mode, it’s important to know that your code is not required to run in strict mode, so you don’t need to rewrite your existing jQuery code if you want to migrate to jQuery 3. Strict & non-strict mode JavaScript can happily coexist.
There’s one exception: some versions of ASP.NET that – because of the strict mode – are not compatible with jQuery 3. If you’re involved with ASP.NET, you can have a look at the details here in the docs.
3. For…of Loops is Introduced
jQuery 3 supports the for…of statement, a new kind of for
loop, introduced in ECMAScript 6. It gives a more straightforward way to loop over iterable objects, such as Arrays, Maps, and Sets.
In jQuery, the for...of
loop can replace the former $.each(...)
syntax, and can make it easier to loop through the elements of a jQuery collection.
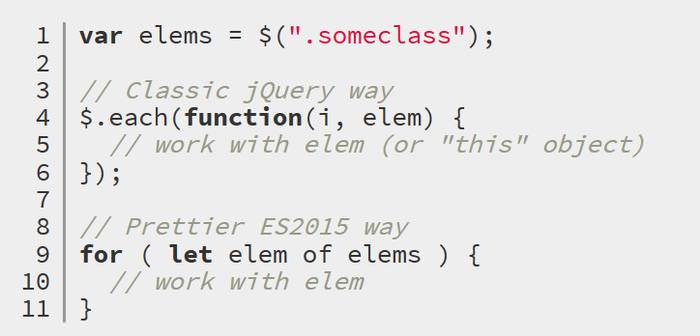
Note that the for...of
loop will only work either in an environment that supports ECMAScript 6, or if you use a JavaScript compiler such as Babel.
4. Animations Got a New API
jQuery 3 uses the requestAnimationFrame() API for performing animations, making animations run smoother and faster. The new API is only used in browsers that support it; for older browsers (i.e. IE9) jQuery uses its previous API as a fallback method to display animations.
RequestAnimationFrame has been out for a while, if you are interested in what it knows or why you should use it, CSS Tricks has a good post about it.
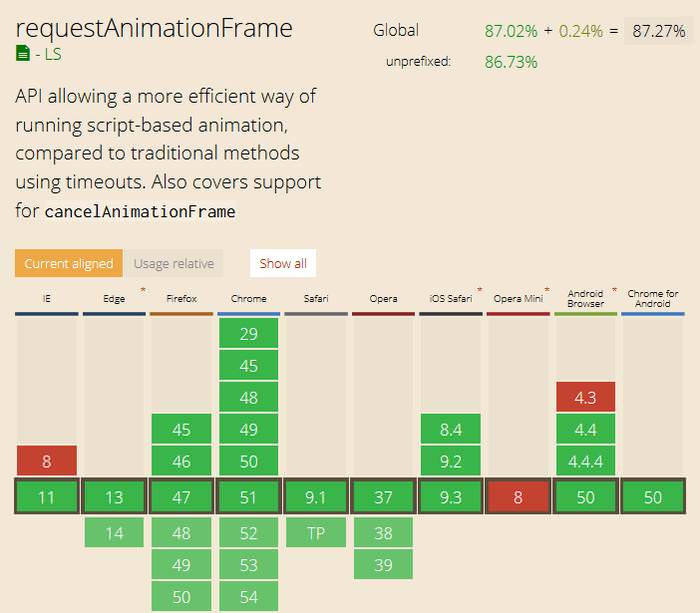
5. New Method for Escaping Strings with Special Meaning
The new jQuery.escapeSelector()
method allows you to escape strings or characters that mean something else in CSS in order to be able to use it in a jQuery-selector; without escaping the JavaScript interpreter cannot properly understand it.
The docs helps us understand this method better with the following example:
“For example, if an element on the page has an id of “abc.def” it cannot be selected with $( "#abc.def" )
because the selector is parsed as “an element with id ‘abc’ that also has a class ‘def’. However, it can be selected with $( "#" + $.escapeSelector( "abc.def" ) )
.”
I’m not sure how frequently such a case happens, but if you bump into a problem like this, now you have an easy way to quickly fix it.
6. Class Manipulation Methods Support SVG
Unfortunately, jQuery 3 still doesn’t fully support SVG, but the jQuery methods that manipulate CSS class names, such as .addClass()
and .hasClass()
, now can be used to target SVG documents as well. This means you can modify (add, remove, toggle) or find classes with jQuery in Scalable Vector Graphics, then style the classes with CSS.
Recommended Reading: Styling Scalable Vector Graphic (SVG) with CSS
7. Deferred Objects Are Now Compatible with JS Promises
JavaScript Promises – objects used for asynchronous computations – have been standardized in ECMAScript 6; their behaviour and features are specified in the Promises/A+ standards.
In jQuery 3, Deferred objects
have been made compatible with the new Promises/A+ standards. Deferreds are chainable objects that make it possible to create callback queues.
The new feature changes how asynchronous callback functions are executed; Promises allow developers to write asynchronous code that is closer in logic to synchronous code.
See code examples from the Upgrade Guide or, have a look at this great Scotch.io tutorial about the basics of JavaScript Promises.
8. jQuery.when() Interprets Multi-Arguments Differently
The $.when()
method provides a way to execute callback functions. It’s part of jQuery since version 1.5. It’s a flexible method; it also works with zero arguments, and it can accept one or more objects as arguments as well.
jQuery 3 changes the way how arguments of $.when()
are interpreted when they contain the $.then()
method with which you can pass additional callbacks as arguments to the $.when()
method.

In jQuery 3, if you add an input argument with the then()
method to $.when()
, the argument will be interpreted as a Promise-compatible "thenable".
This means that the $.when
method will be able to accept a boarder range of inputs, such as native ES6 Promises and Bluebird Promises, that makes it possible to write more sophisticated asynchronous callbacks.
9. New Show/Hide Logic
In order to increase compatibility with responsive design, the code related to showing and hiding elements has been updated in jQuery 3.
From now on, the .show()
, .hide()
, and .toggle()
methods will focus on inline styles, instead of computed styles, this way they will better respect stylesheet changes.
The new code respects the display
values of stylesheets whenever it’s possible, which means that CSS rules can dynamically change upon events such as device reorientation and window resize.
The docs asserts that the most important result will be:
"As a result, disconnected elements are no longer considered hidden unless they have inline display: none;
, and therefore .toggle()
will no longer differentiate them from connected elements as of jQuery 3.0."
If you want to better understand the results of the new show/hide logic, here is an interesting Github discussion about it.
jQuery developers also published a Google Docs table about how the new behaviour will work in different use cases.
10. Extra Protection Against XSS Attacks
jQuery 3 added an extra security layer against Cross-Site Scripting (XSS) attacks by requiring developers to specify dataType: "script"
in the options of the $.ajax()
and the $.get()
methods.
In other words, if you want to execute cross-domain script requests, you must declare this in the settings of these methods.
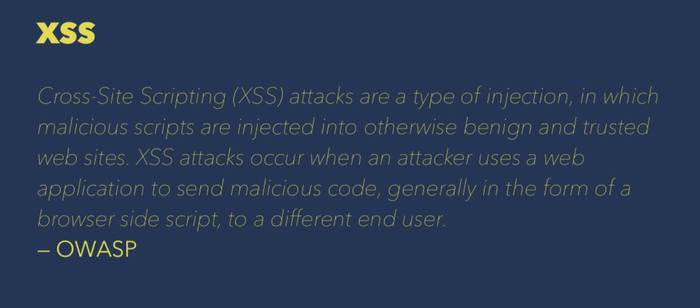
According to the docs, the new requirement is useful when a "remote site delivers non-script content but later decides to serve a script that has malicious intent". The change doesn’t affect the $.getScript()
method, as it sets the dataType: "script"
option explicitly.