Enhance Your WordPress Site with Category and Tag Thumbnails
Enhancing your WordPress site by adding images next to categories or tags can make it more visually appealing. For instance, consider displaying the CSS logo next to the “CSS” category or the HTML5 logo for “HTML” (as shown below).
Since version 2.9, WordPress has supported the Featured Image functionality for posts, pages, and custom post types. Originally termed Image Thumbnail, this feature does not extend to categories, tags, or custom taxonomies, with the possible exception of custom taxonomies in future updates.
With the help of the Taxonomy Thumbnail plugin, we can easily add images to our categories and tags. Let’s explore how to implement this feature using a simple code snippet.
Setting Up Your Plugin
To get started, first install the plugin on your WordPress site. You can add the plugin either directly via Plugins > Add New in your dashboard or by using FTP. After activation, navigate to Post > Categories. Here, you’ll see an option to “Set a thumbnail“.
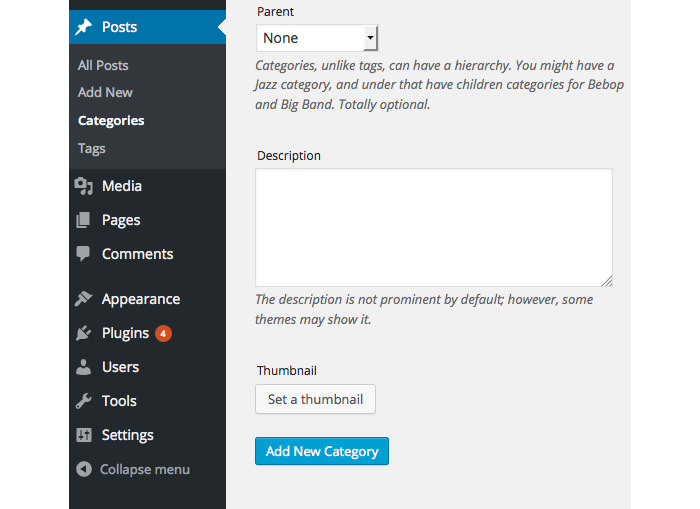
Clicking this button opens the WordPress Media Manager. In this interface, you can either choose a previously uploaded image or upload a new one to customize and set as your category or tag thumbnail.
The selected image will appear in the Category table, providing a visual reference for each category with an associated image.
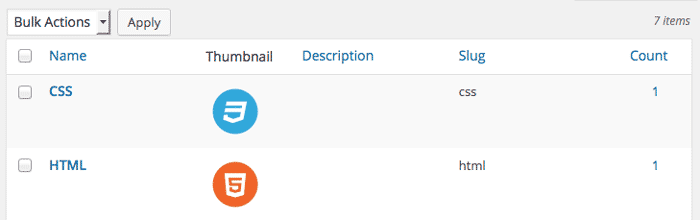
Template Tags Explained
This plugin includes several template tags that function similarly to the Post Thumbnail tags, allowing you to easily retrieve thumbnails for terms.
get_term_thumbnail_id( $term_taxonomy_id )
: Retrieves the thumbnail ID for a taxonomy term.has_term_thumbnail( $term_taxonomy_id )
: Checks whether a taxonomy term has a thumbnail.get_term_thumbnail( $term_taxonomy_id, $size = 'post-thumbnail', $attr = '' )
: Retrieves the thumbnail image for a taxonomy term.
The functions mentioned require a taxonomy ID – whether for a category, tag, or custom taxonomy – which you can find using the term_taxonomy_id
function. The plugin also includes additional functions to set and delete thumbnails, though the ones listed here should cover basic needs.
Displaying Thumbnails
Retrieving Terms
Begin by using the get_terms()
function to retrieve a list of terms from the specified taxonomy-here, we’ll focus on post categories.
<?php $taxonomy = 'category'; $args = array( 'orderby' => 'name', 'order' => 'ASC', 'hide_empty' => true, 'exclude' => array(), 'exclude_tree' => array(), 'include' => array(), 'number' => '', 'fields' => 'all', 'slug' => '', 'parent' => '', 'hierarchical' => true, 'child_of' => 0, 'childless' => false, 'get' => '', 'name__like' => '', 'description__like' => '', 'pad_counts' => false, 'offset' => '', 'search' => '', 'cache_domain' => 'core', ); $terms = get_terms($taxonomy, $args); ?>
This produces an array with details for each term such as term_id
, name
, slug
, and more. To display these terms, use a foreach
loop as shown below:
<?php if (!empty($terms) && !is_wp_error($terms)) { echo '<p>'. $taxonomy .':</p>'; echo '<ul>'; foreach ($terms as $term) { echo '<li>' . $term->name . '</li>'; } echo '</ul>'; } ?>
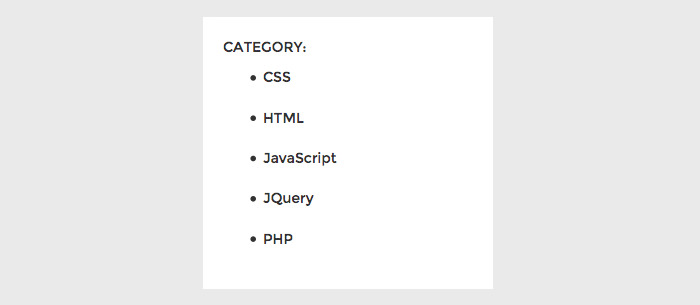
This results in a list of categories such as CSS, HTML, JavaScript, jQuery, and PHP, each potentially with its corresponding image (logo or icon). Now, let’s see how to display these images.
Displaying Thumbnails
To include the thumbnail images, enhance the foreach
loop from the previous code snippet:
if (!empty($terms) && !is_wp_error($terms)) { echo '<ul>'; foreach ($terms as $term) { echo '<li><a href="/index.php/' . $taxonomy . '/' . $term->slug . '">' . $term->name . get_term_thumbnail($term->term_taxonomy_id, $size = 'category-thumb', $attr = '') . '</a></li>'; } echo '</ul>'; }
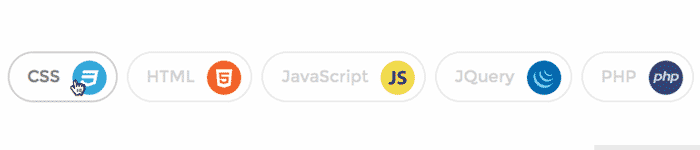
The plugin also allows filtering terms to retrieve only those with thumbnails by using the following parameter in the get_terms()
function:
$taxonomy = 'category'; $args = array( 'with_thumbnail' => true, // true = retrieve terms that have thumbnails, false = retrieve all terms ); $terms = get_terms($taxonomy, $args);
Extending to Other Taxonomies
Similarly, you can apply this functionality not only to categories but also to other taxonomies such as Tags, Link Categories, and Custom Taxonomies. This versatility makes the plugin incredibly useful for enabling image thumbnails across various taxonomies.
Note: This post was first published on the Nov 23, 2015.