How to Dynamically Add and Remove HTML Classes with jQuery
Adding a new HTML class manually is straightforward; however, creating an interactive website requires the ability to modify, insert, and remove HTML classes dynamically. jQuery makes this easy. Below is an example demonstrating how to add and remove a class named my-new-class
from a <div>
.
// Add class $('div').addClass('my-new-class'); // Remove class $('div').removeClass('my-new-class');
Beyond jQuery, you can also manage HTML classes using vanilla JavaScript:
// Add class document.getElementById('elem').className = 'my-new-class'; // Remove class document.getElementById('elem').className = document.getElementsByTagName('div')[0].className.replace(/(?:^|\s)my-new-class(?!\S)/g, '');
Although direct JavaScript manipulation is less succinct than jQuery, it’s a viable option for those preferring not to use a framework. Additionally, the modern classList
API offers an even more convenient method for class manipulation.
How to Get User Location with HTML5 Geolocation API
In many cases, obtaining user location would be extremely useful to establish better user experience, for example: E-commerce... Read more
Manipulating Classes with the classList API
The classList
API, much like jQuery, provides methods for easy modification of HTML classes.
For a div
containing multiple classes, classList
allows retrieval of all classes:
var classes = document.getElementById('elem').classList; console.log(classes);
Accessing the browser Console will display the list of classes.
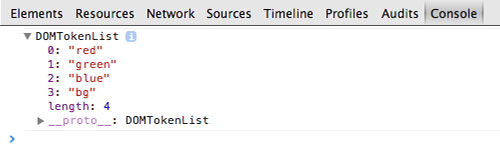
To modify classes, utilize .add()
and .remove()
methods:
var elem = document.getElementById('elem'); // Add class elem.classList.add('my-new-class'); // Remove class elem.classList.remove('my-new-class');
Adding multiple classes is straightforward, simply separate each class with a comma:
elem.classList.add('my-new-class', 'my-other-class');
To verify the presence of a specific class, .contains()
returns true if found, and false otherwise:
elem.classList.contains('class-name');
Class toggling based on user interaction, such as a mouse click, is achieved with .toggle()
:
var button = document.getElementById('button'); function toggle() { elem.classList.toggle('bg'); } button.addEventListener('click', toggle, false);
Explore the functionality through these links:
Concluding Thoughts
The classList
API, a sleek addition with HTML5, simplifies class manipulation in modern browsers such as Firefox 3.6, Opera 11.5, Chrome 8, and Safari 5.1. However, it’s not supported in Internet Explorer 9 and earlier. For compatibility in these cases, consider using Polyfills to implement the classList API in Internet Explorer.
Additional Resources
- The classList API — HTML5 Doctor
- Element classList — MDN
Note: This post was first published on January 17, 2014.