How to Remove Unnecessary CSS Using Grunt
Using a framework like Bootstrap and Foundation is one of the fastest ways to build a responsive website. These frameworks include everything you need, such as the Grid and User Interface components, to create a functional and attractive site.
However, these frameworks are designed to cover a wide range of scenarios, so they come with lots of predefined CSS that you might not need for your website.
Not every style is necessary for your specific needs, and having all these unused styles increases your stylesheet size, which can negatively impact your website’s loading performance. In this article, we’ll show you how to remove those unnecessary styles from your stylesheets using Grunt.
Quickly Fix Grunt ‘Command Not Found’ Error in Terminal
In one of our previous post we have discussed how to build customized jQuery. In the process, we... Read more
Getting Started
To achieve this, we need Grunt CLI. Make sure you have it installed on your computer. You can refer to these previous articles for more information:
Assuming you have a project directory ready with HTML and CSS files, let’s proceed.
As shown in the screenshot below, I have two folders: build (for saving stylesheets during development) and css (for the final output of the stylesheet).
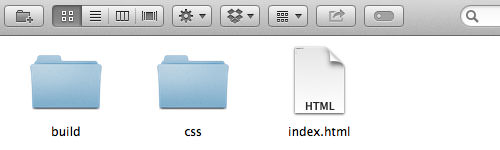
Navigate to your project directory through Terminal and enter the following commands. These commands install Grunt and grunt-uncss, the Grunt plugin needed to remove unused CSS.
npm install grunt --save-dev npm install grunt-uncss --save-dev
Basic Configuration
Create a file named Gruntfile.js in your project directory. Open it in a code editor and add the following code:
module.exports = function(grunt) { grunt.initConfig({ uncss: { dist: { files: { 'css/style.css': ['index.html'] } } } }); grunt.loadNpmTasks('grunt-uncss'); grunt.registerTask('default', 'uncss'); };
This basic configuration of grunt-uncss
fetches the stylesheet links from the .html
file, identifies unused CSS selectors, and outputs the cleaned CSS to css/style.css
.
Optional Configuration
The grunt-uncss
plugin offers several options. For instance, if you want to ignore certain selectors, you can use the ignore
parameter:
module.exports = function(grunt) { grunt.initConfig({ uncss: { dist: { files: { 'css/style.css': ['index.html'] } }, options: { ignore: ['#id-to-ignore', '.auto-generated-class', '.ignore-this-class'] } } }); grunt.loadNpmTasks('grunt-uncss'); grunt.registerTask('default', 'uncss'); };
You can also specify particular stylesheets to process using the stylesheets
parameter:
module.exports = function(grunt) { grunt.initConfig({ uncss: { dist: { files: { 'css/style.css': ['index.html'] } }, options: { ignore: ['#id-to-ignore', '.auto-generated-class', '.ignore-this-class'], stylesheets: ['build/style.css'] } } }); grunt.loadNpmTasks('grunt-uncss'); grunt.registerTask('default', 'uncss'); };
Adjust the output path according to your project’s requirements.
Run UnCSS
Here’s the content of my HTML file:
<html> <head> <title>CSS Unloaded</title> <link rel="stylesheet" href="//cdnjs.cloudflare.com/ajax/libs/normalize/3.0.0/normalize.min.css"> <link rel="stylesheet" href="css/style.css"> </head> <body> <div class="wrapper"> <h1 class="page-title">Unload CSS</h1> <div class="first-div"><p>1st</p></div> <div class="second-div"><p>2nd</p></div> <div class="third-div"><p>3rd</p></div> </div> </body> </html>
And here is the content of my stylesheet:
.wrapper { width: 960px; margin: 60px auto; } .page-title { color: red; } .first-div, .second-div, .third-div, .fourth-div { width: 100px; height: 100px; } .first-div { background-color: #000; color: #fff; } .second-div { background-color: #555; color: #ccc; } .third-div { background-color: #ccc; color: #f3f3f3; } .fourth-div { background-color: #aaa; color: #777; position: absolute; }
The only class not present in the HTML file is .fourth-div
. This class will be removed from the stylesheet. Launch Terminal and type grunt
to run the task configured in Gruntfile.js.
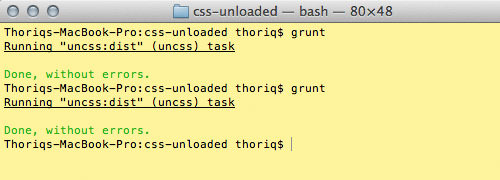
Open the CSS files. You’ll see that the .fourth-div
class selector has been removed since it’s not used in the HTML.
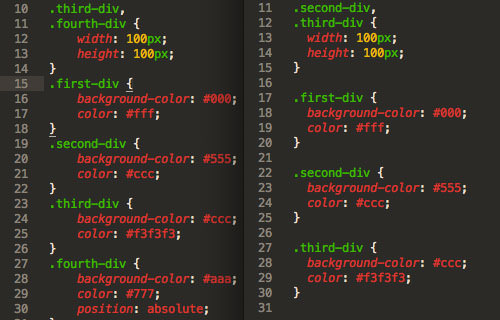
If you have many unused styles, this method can help you significantly reduce your stylesheet file size.