Learning JavaScript: Things to Know Before You Start
There is no doubt that JavaScript is an extensive programming language with plenty of helper libraries, frameworks, databases, and other extra functionalities. The piling up of new information can be intimidating for the novice programmer. As Kyle Simpson says in “You Don’t Know JavaScript”: “You write the code in JS first, and then you find out how it works.“
I’ve worked on projects built in both pure JS and have also worked with C# for several years. In my spare time, I also coach and teach new developers and give presentations at IT conferences. And based on my experience, I am going to help you figure out how to learn it.
In this article, I’d like to highlight the things that the novice JS learners need to know at the very begining of the learning process. These tips could ease the leaning curve and may even make it interesting for you to start into JS
So, let’s move forward.
JavaScript Jargon: 10 Terms You Should Know
From currying to closures there are quite a number of JavaScript jargons (special words used within the field)... Read more
Gradually educate yourself
At first sight, a developer’s roadmap will show you how extensive the JS ecosystem is and how much you have to understand. Seeing such an array of information, many novice developers trap themselves and mistakenly think they need to know it all at once.
They try to make sense of the entire JS ecosystem without delving into JS itself. They end up understanding a lot of stuff on the surface as they hop between topics. These developers will not become specialists in any field if they take this strategy.
Despite the fact that JavaScript is a fast-evolving and expanding programming language, it is based on a number of fundamental ideas and underlying themes. Any project of any complexity can be facilitated using these methods.
From my perspective, a roadmap for a new JS developer should look like this:
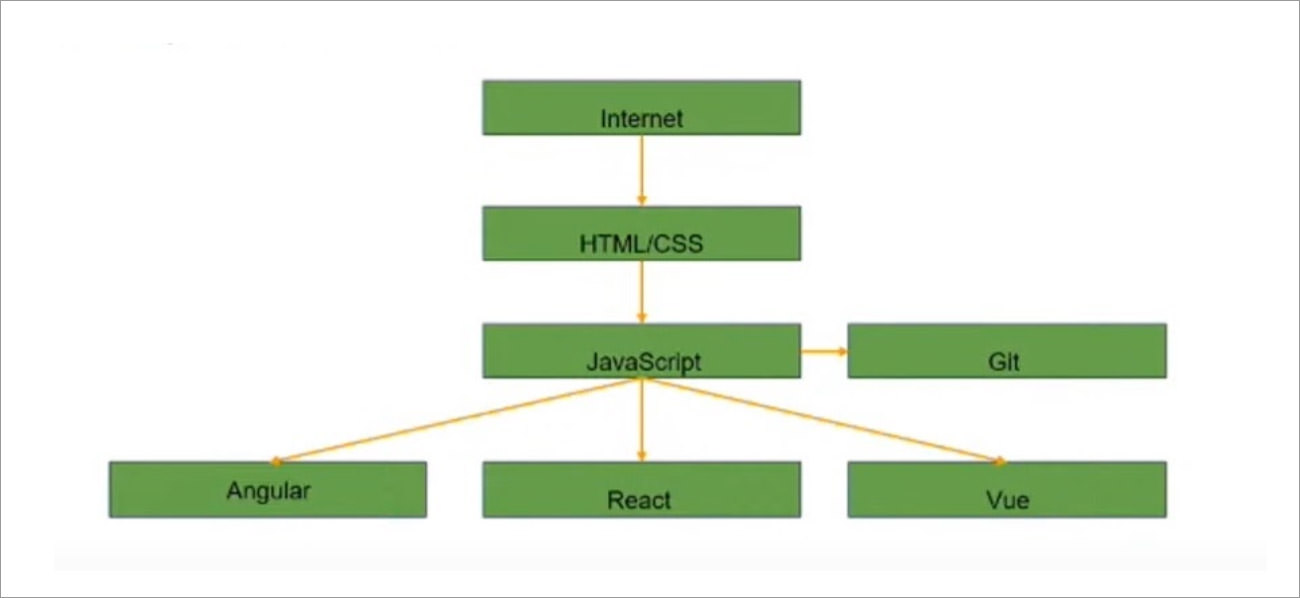
This is the method we use to understand the fundamentals:
- How the Internet works – I don’t think I need to explain why you need to know this
- Layout – yes, the JS-developer must deal with this on a frequent basis as well
- Basic JS – you should understand not just how to declare a variable, but also all of the language’s nuances
- Git – working on projects without a proper understanding of Git can lead to significant time wasted
- S framework – I would start with React, but I recommend looking through all of the options and selecting the most appealing one. Of course, there are more on the way.
Every stage in this process is supported by a massive amount of data. Determine the distinctions between client and server, delve into HTML and CSS, and decide which JS framework (Angular, React, or Vue) best suits your professional goals, and begin learning wisely.
This expertise will boost your chances of landing a job. Also, a solid mastery of the fundamentals of JavaScript will stick with you for the rest of your life. As a result, the first rule is to approach JavaScript methodically, rather than scattering your attention with the aim of learning everything at once.
Delve deep into the basic topics
You can find bottlenecks even in the basic themes that are not immediately noticeable, but which become apparent in the code of your actual project. And at this point, you need to grasp what’s going on and how to deal with it. Here’s a great example of an interview question for a newbie:
const resultA = 40 && 2 || 33; const resultB = (4 * 2) && (3 * 6); const resultC = 0 && 2
Often half of the novice developers make mistakes when answering the question of what will happen with variables a
, b
, c
. Many believe that boolean values should be returned here – true
or false
.
But in this code, if all values are true
, the last true value will be returned, or the first false
value, if at least one of them is false. In our case, 2
, 18
, 0
, respectively. Here is another example of the most mainstream task from the JS world:
for (var i = 0; i < 10; i++) { setTimeout ( function () { console.log(i); } , 1000) ;
If you fully grasp just the concepts in this example, you will also understand:
- Areas of visibility
- Closures
- The difference in how variables work
- Popping up variables
- How setTimeout and asynchronous JS work.
This leads to the second rule: while interpreting such cases, investigate deeper into the answers provided.
Beware of “standards”
On various resources, there is frequently a distinction between “old JS standard” and “new JS standard.” Features from ES6, ES7, and the following versions of JavaScript are allegedly new tools that may be learned by becoming part of a team.
Beginning programmers have the incorrect belief that they can be learned separately, however, this is not the case. Destructuring, arrow functions, Spread-operators, promises, and classes are all features that have long been used as a modern language standard. It is critical to understand how to work with them.
Without practice, JS is like a leaf in the wind
Assume you aced your first interview for a Junior JavaScript Developer position and showed exceptional theoretical comprehension. You are employed for an internship in the team and given your first task.
Then you find you can’t write a single line of code by yourself! Now is a good moment to mention a third crucial rule: put your theoretical knowledge into practice at all times. You will not only learn how to perform application tasks rapidly but also how to navigate into the fundamental JavaScript principles, through rigorous practice. This is a useful skill for potential customers.
Try to tackle the challenge, which you may meet in any test task. There is a list of objects, each with its unique price. Output to the user the final total of the goods in the shopping cart that has been selected.
const goodsAddedToCart - [ { name: 'JavaScript' , price: 20 } , { name: 'React' , price: 30 } , { name: 'HTML/CSS' , price: 202 } ] ;
I’m sure many newbies will easily describe the solution by creating the for
loop and the result
variable with a null value assigned to it. It doesn’t look very elegant, but it works, right?
var result = 0; for (i = 0; i < goodsAddedToCart.length; i++) { result = result + goodsAddedToCart[i].price; } ;
But how can we reuse this method for a different sequence of selected goods – apparently it all has to be wrapped into a function, but why would we do that when JS has been providing tools for such tasks for a long time, here’s an example with reducing:
goodsAddedToCart.reduce ( (accumulator, ( price } ) => accumulator + price, 0);
One more example:
const getAllPeople = async () => { const response = await fetch('https://swapi.dev/api/people/'}; const result = await response.json( ); return result.results; } ; const displayPersonData = ( person, elementToDisplay) => { const personWrapper = document.createElement ( 'div') ; elementToDisplay. appendChild (personWrapper) ; personWrapper. style. margin = '10 px' ; for (let [key, value] of Object. entries (person)) { const personInfoField = personWrapper. appendChild (document createElement( 'div')) ; personInfoField.innerHTML = 'S { key} : $ {value} : ' ; } const displayPeople = async ( ) => { const people = await getAllPeople( ) ; const documentBody = document.querySelector( 'body' ) ; document.Body.innerHTML = ' ' ; people. forEach ( (person) => { displayPersonData(person, documentBody) ; } ) ; } ;
Of course, this code can be enhanced further. Even so, it is sufficient to practice working with searches, arrays, and objects. There are numerous free APIs available on the Internet that will allow you to practice dealing with this type of functionality. For instance, consider the following resources:
- The Star Wars API: SWAPI
- alexwohlbruck/cat-facts
- jikan.docs.apiary.io
- Google.
The importance of concise and clear code
Another blunder made by Juniors is failing to name variables in their code. The main requirement for being understood by other programmers on your team or after a few months of pause, including yourself, is readability. As an example, consider an array of users, each with a name and an age:
const users = [ { name: 'John' , age: 20 } , { name: 'Alex' , age: 30 } , { name: 'Thanos' , age: 32432 } ] ;
Imagine you used the Map processing method when working with this array, which would make sense, but for some reason, you named the parameter of the anonymous function as itm:
users.map ( (itm) => { // There are 100 lines of code } ) ;
Why should this be considered as an error in this context, when everything will work with this or another variable name? Of course, the error here is logical rather than programmatic. Why not call each element of an array “user” if you’re working with an array of data users?
This way, you won’t get angry feedback from other engineers who just don’t comprehend what this or that variable in your code performs.
Here’s another example of code bloat:
const getPersonAppearance = (object) => { const personShortDescription = object. name + " " + object. surname + " in his " + object. age + "looks awesome"; // There are 100 lines of code return { personShortDescription, ... } };
By simply destructuring the array objects (name, surname, age) you can get a concise and understandable to every programmer text:
const getPersonAppearance = ( person ) => { const { name, surname, age} = person; const personShortDescription = ' S {name} S {surname} in his {age} looks awesome ' ; // There are 100 lines of code return { personShortDescription, ... } };
So here is the fourth rule: by developing good habits during training, you will not have to adjust them in the workplace.
Be a learner
It is critical in programming to never end there. The IT industry is rapidly evolving, with the appearance of new frameworks and measures for working with data.
If you don’t gradually master them, you risk becoming mired down in repetitive and monotonous chores for an extended period of time. That’s not why you got into the profession, is it?
Once you have fundamental JS programming skills, give yourself a pat on the back, take a deep breath, and return to studying a full-fledged JavaScript roadmap.