How to Use HTML5 Fullscreen API
We have always been able to view a whole webpage in fullscreen mode. To do so, you can hit F11 key in Windows, while in OS X you can hit Shift + Command + F. However, there are times when we, as web developers, want to add a trigger on the webpage to perform the function rather than relying on those keys.
In addition to providing several new elements, HTML5 also introduced a set of new APIs, including one we are going to discuss in this post: the Fullscreen API. This API allows us to put our website, or just a particular element on the webpage, into fullscreen mode (and vice versa) using the browser’s native functionality.
As far as implementation is concerned, this API would be useful particularly for videos, images, online games, and HTML/CSS-based slide presentations.
So, let’s see how it works.
Recommended Reading: More HTML5 / CSS3 Tutorials
Browser Support
At the time of writing, this API only works for Google Chrome, Safari, and Firefox. Similar to CSS3, the syntax is prefixed as it is still in an experimental stage.
Webkit | Firefox | Description |
---|---|---|
webkitRequestFullScreen |
mozRequestFullScreen |
The method to send the webpage or specified element fullscreen. |
webkitCancelFullscreen |
mozCancelFullscreen |
The method to exit fullscreen mode. |
mozFullScreenElement |
webkitFullScreenElement |
The method to check whether the element is in fullscreen mode. |
It is worth noting that the Fullscreen API is subject to change in the future.
Usage Example
One of the best ways to learn something new is by example. In this post, we will run a simple project. The idea is to have an image and a button that will put the image fullscreen or change it back to normal view with a click.
HTML
Let’s start with the HTML markup. We have a <div>
element to wrap the image and a <span>
element for the button.
<div id="fullscreen" class="html5-fullscreen-api"> <img src="img/arokanddedes.jpg"> <span class="fs-button"></span> </div>
CSS
Then, we place the image at the center of the window and add a few decorative styles to make it look nicer.
.demo-wrapper { width: 38%; margin: 0 auto; } .html5-fullscreen-api { position: relative; } .html5-fullscreen-api img { max-width: 100%; border: 10px solid #fff; box-shadow: 0px 0px 50px #ccc; } .html5-fullscreen-api .fs-button { z-index: 100; display: inline-block; width: 32px; height: 32px; position: absolute; top: 10px; right: 10px; cursor: pointer; }
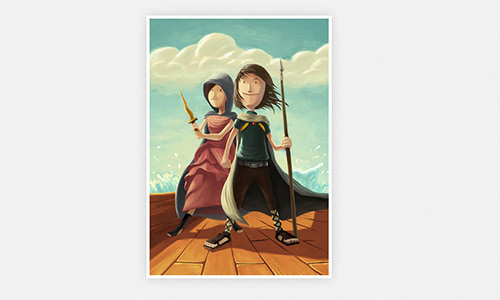
I decided to display the icon in the <span>
element using the :after
pseudo-element, so that later we can change the icon easily through CSS using the content
attribute.
.html5-fullscreen-api .fs-button:after { display: inline-block; width: 100%; height: 100%; font-size: 32px; font-family: 'ModernPictogramsNormal'; color: rgba(255,255,255,.5); cursor: pointer; content: "v"; } .html5-fullscreen-api .fs-button:hover:after { color: rgb(255,255,255); }
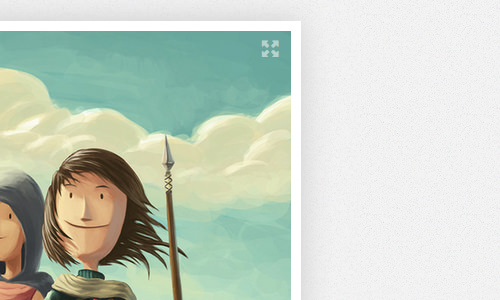
JavaScript
We will use jQuery to make the code a little leaner.
As mentioned, we will send the image fullscreen upon a click. We wrap the function under the jQuery .on
method.
$('.fs-button').on('click', function(){ });
First, we check whether the element is already in fullscreen mode. If this condition returns true, we will execute webkitCancelFullScreen
to return it to the normal view. Otherwise, we will turn it fullscreen using the webkitRequestFullScreen
method, like so:
$('.fs-button').on('click', function(){ var elem = document.getElementById('fullscreen'); if(document.webkitFullscreenElement) { document.webkitCancelFullScreen(); } else { elem.webkitRequestFullScreen(); }; });
Click on the fullscreen icon, and our image will go fullscreen, as shown in the following screenshot.
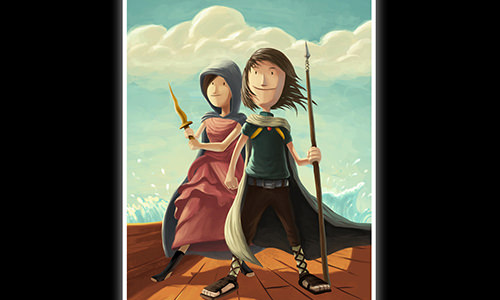
Fullscreen CSS
Webkit (as well as Firefox) also provides a set of new pseudo-classes that allow us to add style rules when the element is in fullscreen mode. Say we want to change the background; we can write the style rules this way:
#fullscreen:-webkit-full-screen { background-image: url('../img/ios-linen.jpg'); width: 100%; }
Now, you should see the iOS linen texture in fullscreen mode.
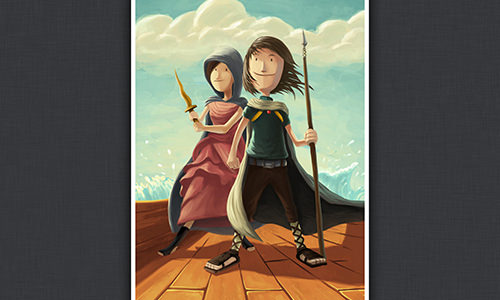
That’s it. You can head over to the demo page to see it in action. Since we do not specify the function with the Firefox syntax, this demo will only work in Webkit Browsers: Google Chrome and Safari.
Further References
- Fullscreen API Draft — W3C
- Using Full Screen Mode — MDN
- HTML5 Fullscreen API — Sitepoint