Six Essential jQuery Practices to Boost Performance
Editor’s note: This article is part of our Code Optimization series, where we explore techniques to optimize code for better efficiency and improve our coding skills.
jQuery remains one of the most popular JavaScript libraries today, thanks to its user-friendly API and relatively shallow learning curve. Many developers opt to use jQuery instead of vanilla JavaScript to introduce dynamic functionalities to their projects.
However, jQuery is not without its drawbacks. If not used correctly, it can lead to performance issues, much like the JavaScript language it is built upon. This post outlines best practices for using jQuery effectively to help you avoid potential performance pitfalls.
1. Lazy load scripts only when necessary
Browsers execute JavaScript before fully creating the DOM tree and rendering the page because scripts can add new elements or alter the layout or style of existing elements. By minimizing the number of scripts the browser has to execute during page load, you can reduce the time it takes for the DOM tree to be created and painted, allowing users to see the page sooner.
In jQuery, you can achieve this by using $.getScript
to load script files as they are needed, rather than during the initial page load.
$.getScript("scripts/gallery.js", callback);
This is an AJAX function that retrieves a single script file when needed. However, note that the fetched file isn’t cached by default. To enable caching for getScript
, you’ll need to enable it for all AJAX requests using the following code:
$.ajaxSetup({ cache: true });
2. Avoid $(window).load()
unless sub-resources are required
The $(document).ready()
function is equivalent to DOMContentLoaded
(where available), and $(window).load()
is equivalent to the Load
event. The former is triggered when the DOM is fully loaded, excluding external assets like images and stylesheets. The latter is triggered only after all page resources, including external assets, are fully loaded.
Use $(window).load()
if your script depends on sub-resources of the page, such as changing the background color of a div
that is styled by an external stylesheet. Otherwise, it is better to stick with $(document).ready()
as jQuery automatically calls its ready
event handler, regardless of whether you use $(document).ready()
or not.
3. Use detach()
to temporarily remove elements from the DOM
“Reflow” refers to the process where the browser recalculates the layout of the page due to changes in elements, such as adding new elements, adjusting existing ones, or removing elements. Reflows are resource-intensive operations for the browser.
To minimize the number of reflows caused by structural changes, perform changes to an element after removing it from the page flow and then reinserting it once modifications are complete. For example, if you are adding multiple rows to a table, it is better to take the table out of the DOM, make your changes, and then reinsert it.
jQuery’s detach()
method allows you to remove an element from the page while preserving its data for later use. The detached element can then be reinserted into the DOM once modifications are done.
4. Prefer css()
over height()
and width()
for setting dimensions
When setting the height or width of an element in jQuery, it is recommended to use the css()
function. Using height()
and width()
can trigger additional reflows due to the internal function computeStyleTests
that jQuery uses (tested in the latest version).
For instance, setting the height using p.height("300px");
results in more reflows.
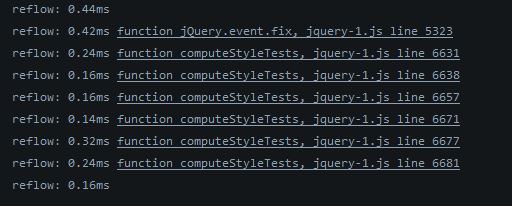
In contrast, using p.css({"height": "300px"});
reduces the reflows.

The computeStyleTests
function performs support tests. It is called when getting the height and width using both css()
and height()/width()
, but for setting, it is only called for height()/width()
, which may be unnecessary. Hence, it is better to use css()
instead.
5. Avoid unnecessary access to layout properties
Accessing layout properties like height, width, or margin can trigger a reflow in the page. This is because, whenever you ask the browser for any layout properties, it ensures you get the most up-to-date values by recalculating them and applying any necessary layout changes.
Whether you are using jQuery or vanilla JavaScript, avoid unnecessary access to layout properties, especially in loops or after making style changes, as this can cause performance issues.
6. Utilize caching wherever possible
jQuery provides several functions that include built-in caching mechanisms, which can be advantageous. While AJAX requests typically cache resources, this does not apply to script
and jsonp
. To enable caching across all AJAX requests, you may want to set it globally as shown earlier.
Also, keep in mind that if you fetch resources using post
, they will not be cached, even if you have caching enabled.
As mentioned earlier, detach()
caches data associated with the element being removed, unlike remove()
. The hide()
function caches an element’s initial display
value before hiding it so it can be restored later without losing data.
Conclusion
To ensure you are using the most efficient jQuery code for your needs, test your code in real scenarios and check for any performance issues. If you notice any, use performance and debugging tools to identify the root cause of the issue.
Since jQuery serves as a wrapper for JavaScript, offering additional functionalities for browser compatibility and other features, it can be challenging to diagnose problems without these tools.